Use Schema Validator to apply JSON Schema, the recommended way of performing MongoDB schema validation to a collection. Get started today.
Add MongoDB schema validation
- Right-click on your target connection (e.g. Customers) in the Connection Tree.
- Choose Add/Edit Validator. This will open the Edit Validator window.
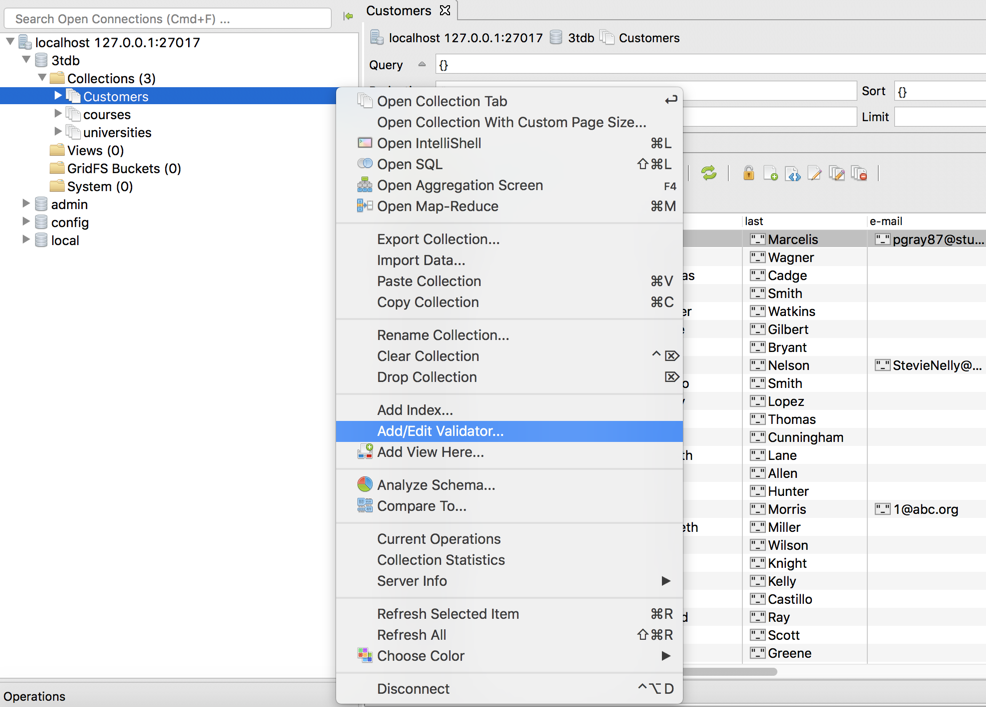
Paste JSON Schema validation rules
In the Edit Validator window, type in or paste your validation rules using JSON Schema.
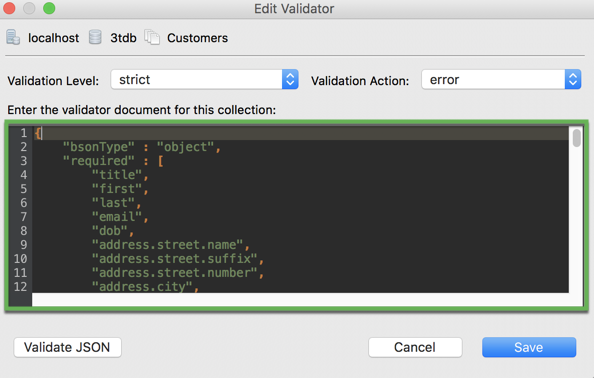
Example of JSON Schema
Below is the JSON Schema we applied to the Customers collection:
{ "bsonType" : "object", "required" : [ "title", "first", "last", "email", "dob", "address.street.name", "address.street.suffix", "address.street.number", "address.city", "address.state", "address.zip-code", "user_name", "package", "prio_support", "registered_on", "transactions", "pet", "number_pets", "Studio_3T_edition", ], "properties" : { "title" : { "bsonType" : "string", "description" : "must be a string and is required" }, "first" : { "bsonType" : "string", "description" : "must be a string and is required" }, "last" : { "bsonType" : "string", "description" : "must be a string and is required" }, "email" : { "bsonType" : "string", "description" : "must be a string and is required" }, "dob" : { "bsonType" : "date", "description" : "must be a date and is required" }, "address.street.name" : { "bsonType" : "string", "description" : "must be a string and is required" }, "address.street.number" : { "bsonType" : "string", "description" : "must be a string and is required" }, "address.street.suffix" : { "bsonType" : "string", "description" : "must be a string and is required" }, "address.city" : { "bsonType" : "string", "description" : "must be a string and is required" }, "address.state" : { "bsonType" : "string", "description" : "must be a string and is required" }, "address.zip_code" : { "bsonType" : "string", "description" : "must be a string and is required" }, "user_name" : { "bsonType" : "string", "description" : "must be a string and is required" }, "package" : { "bsonType" : "string", "description" : "must be a string and is required" }, "prio_support" : { "bsonType" : "string", "description" : "must be a string and is required" }, "registered_on" : { "bsonType" : "date", "description" : "must be a date and is required" }, "transactions" : { "bsonType" : "int32", "description" : "must be an integer and is required" }, "pet" : { "bsonType" : "string", "description" : "must be a string and is required" }, "number_pets" : { "bsonType" : "int32", "description" : "must be an integer and is required" }, "Studio_3T_edition" : { "bsonType" : "string", "description" : "must be a string and is required" } } }
Set validation levels
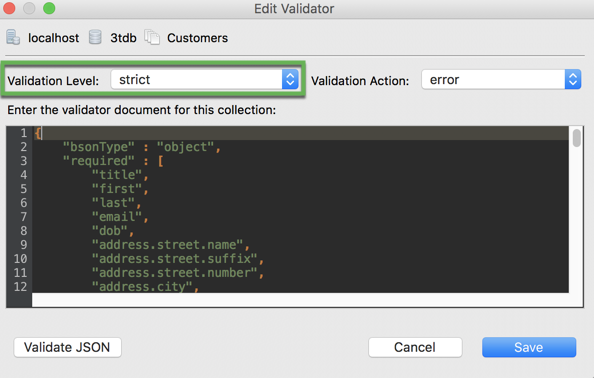
You can choose how MongoDB applies your validation rules:
- off – MongoDB disables validation completely
- strict (default setting) – MongoDB applies validation rules to all inserts and updates
- moderate – MongoDB applies validation rules to inserts and to updates to existing documents that already fulfill the validation criteria
Set validation action
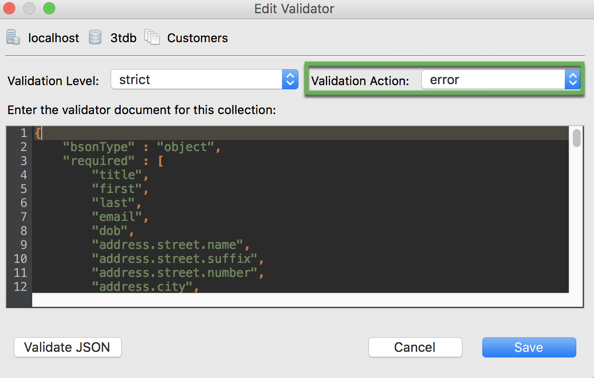
MongoDB can handle documents that don’t meet the validation rules in two ways:
- error (default setting) – Rejects inserts or updates that do not meet validation rules
- warn – Allows inserts and updates while logging violations
Validate and save validation rules
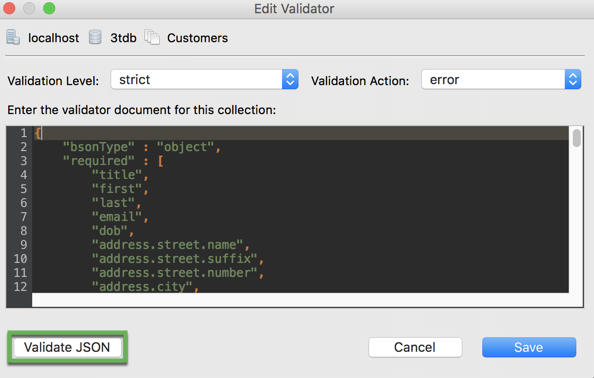
Click on Validate to make sure the JSON is correct, then Save.
JSON Schema validation tutorial
Want to dive deeper into this topic? Read our tutorial, How to Create and Validate JSON Schema in MongoDB Collections, to learn more about how you can use SQL, Schema Explorer, and JSON Schema validation tools to help create your MongoDB schema.