Need to optimize MongoDB queries? Visual Explain is a performance tuning tool that shows the stages MongoDB took to run a query, with runtime statistics. Use Visual Explain in combination with Query Profiler to find slow queries and detect missing indexes so that you can optimize MongoDB performance. Use Index Manager to add indexes, edit indexed fields, and check out index usage.
Try Studio 3T for freePerformance tuning with Visual Explain
Visual Explain is a visual representation of the output from the MongoDB explain () method. With its in-depth view of the MongoDB query plan, Visual Explain helps you to investigate queries and:
- Know when to add an index
- Spot uneven shard loads
- Optimize queries by applying relevant filters first
- Debug queries
Know when to add an index
To illustrate how useful Visual Explain can be, let’s take a look at the titles
collection, which is part of the Pubs database.
It contains 10,000 documents, each containing 12 fields: _id
, title_id
, title
, type
, TypeList
, pub_id
, price
, advance
, royalty
, ytd_sales
, notes
, and pubdate
.
To test how expensive a query can be, let’s run a simple query in Visual Query Builder.
Let’s sort the collection by price in descending order:
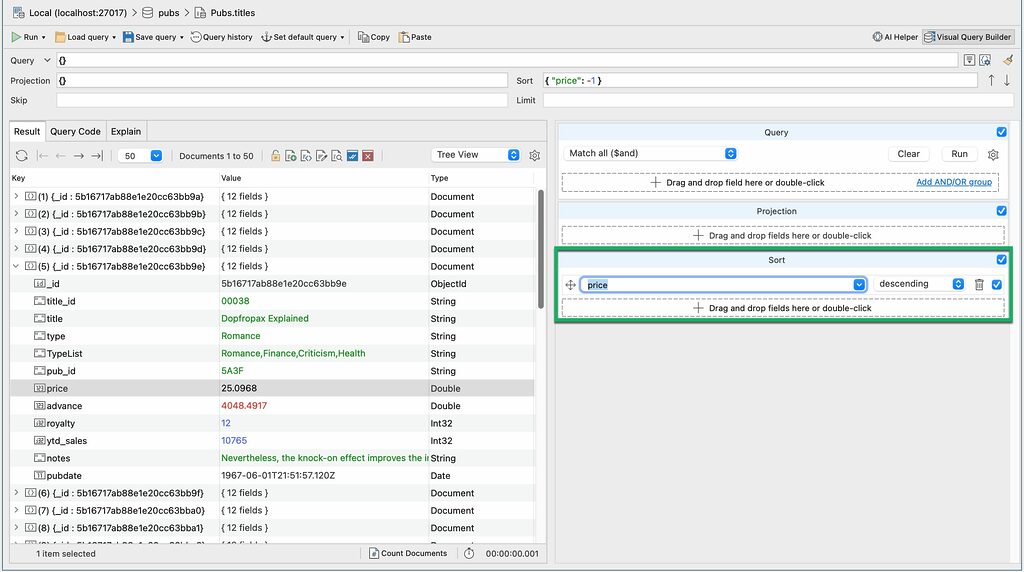
db.getCollection("titles").find({}).sort( { "price" : -1.0 } );
Now, let’s click on the Explain tab.
By default, Studio 3T shows brief mode, a diagram of stages without runtime statistics.
To show runtime statistics in full mode, click on the Run full explain button:
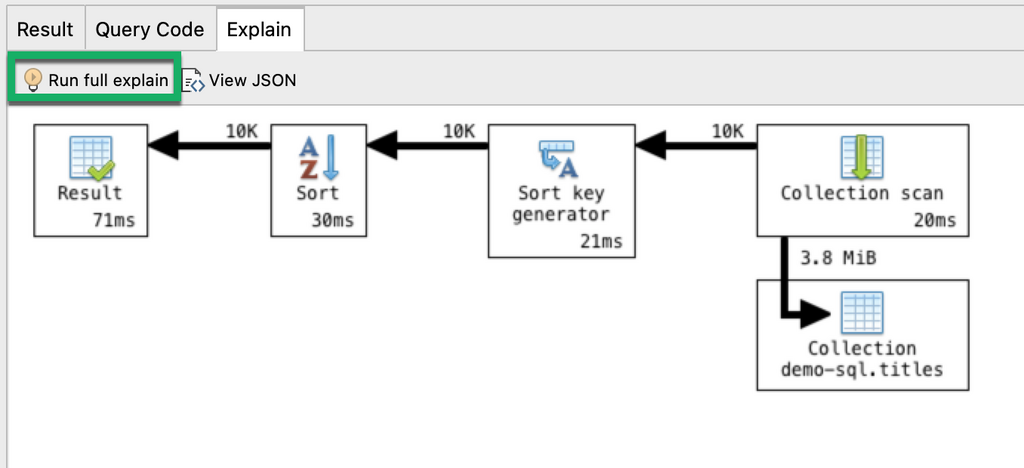
What information can we gather from this?
- It took MongoDB 20 milliseconds to run the collection scan
A collection scan means that the query looked at each document to get the results.
2. 10,000 documents were passed at each stage
3. It took MongoDB 21 milliseconds to run the Sort key generator stage and another 30 milliseconds to run the Sort stage.
4. The whole query took 71 milliseconds to run.
Now, how can we optimize this query?
If you often find yourself sorting price in descending order, then it is best to create an index on the field price
.
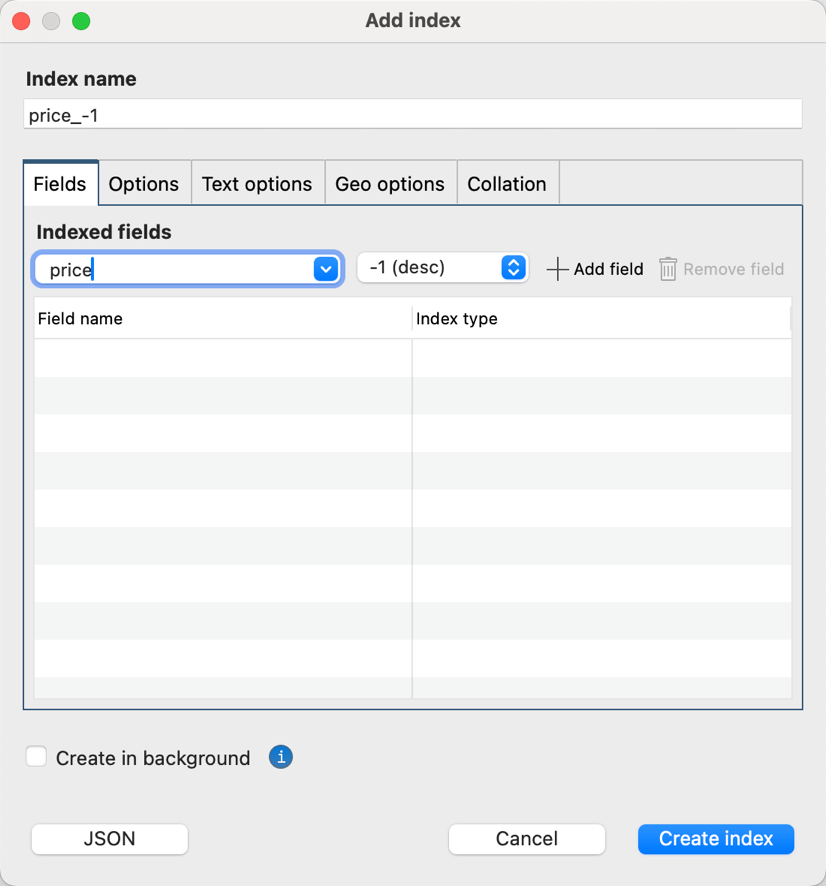
Now that the index is set up, let’s run the same query again (and this time, we expect it to be faster).
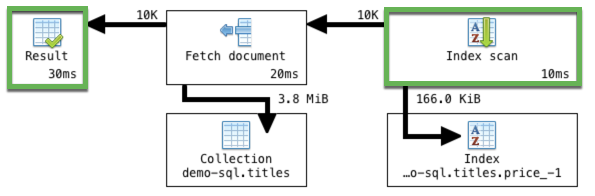
Indeed, MongoDB runs the same query much faster, at 30ms (versus 71ms).
Instead of using a collection scan which took 20ms, MongoDB chose an index scan which only took 10ms. The database detected that an appropriate index exists for the query, so it scanned a limited number of documents, instead of needlessly scanning each one.
By choosing the index scan, the database was also able to skip the sort stages, and instead went straight to fetching the documents, resulting in 41ms saved.
Spot uneven shard loads
MongoDB uses sharding to horizontally scale data, which it tries to evenly distribute across shards – but this is not always the case.
Visual Explain can come in handy for spotting imbalanced loads when you’re querying a sharded database.
Take for example the screenshot below of crimes
, a collection of about 2,486,000 documents, hosted on a database with two shards.
To test, we run an empty query and discover that:
- Shard 1 handled 1.6 million documents
- Shard 2 handled 893K documents
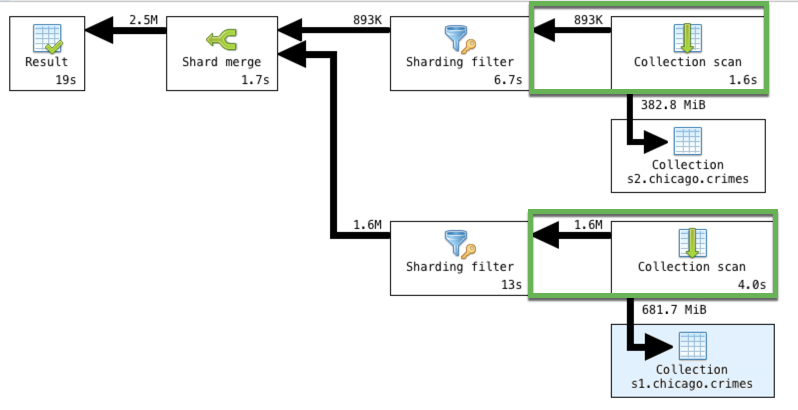
Now we know that the database isn’t distributing the workload evenly as expected. Shard 1 is handling almost twice the load.
We can then move on to the next steps of investigating the sharded cluster balancer or chunk size to fix a bigger issue, which Visual Explain helped us uncover.
Optimize queries by applying relevant filters first
When it comes to querying, the best practice is to apply operators that do the most filtering first.
Visual Explain is an ideal way to check if this is being done.
Using the same crimes
collection from above, we’d like to know how many cases of theft occurred on Chicago’s public transport system, Chicago Transit Authority (CTA).
We’d also like to sort these cases in descending order by date, and we’d only like to see the four relevant fields _id
, location.description
, primary_type
(of crime), and date
.
Here’s the full query that was run in Visual Query Builder:
db.getCollection("crimes").find( { "location.description" : /.*cta.*/i, "primary_type" : "Theft" }, { "_id" : 1.0, "location.description" : 1.0, "primary_type" : 1.0, "date" : 1.0 } ).sort( { "date" : -1.0 } );
Now let’s click on the Explain tab.
What we see is exactly what we want to happen: the database is applying the filter operator first.
From the original 2.5M documents, only 13K meet the filter criteria and are then passed on to the later stages.

If Visual Explain shows you that this isn’t the case, then the next step would be to debug your query.
A way to debug
Say, for example, that you wanted to know how many customers have priority support and live in Kansas, from your pool of 1,000 customers.
You already have a hunch that this can’t be a big number, but Visual Explain shows that 485 documents – 485 customers – meet the criteria:
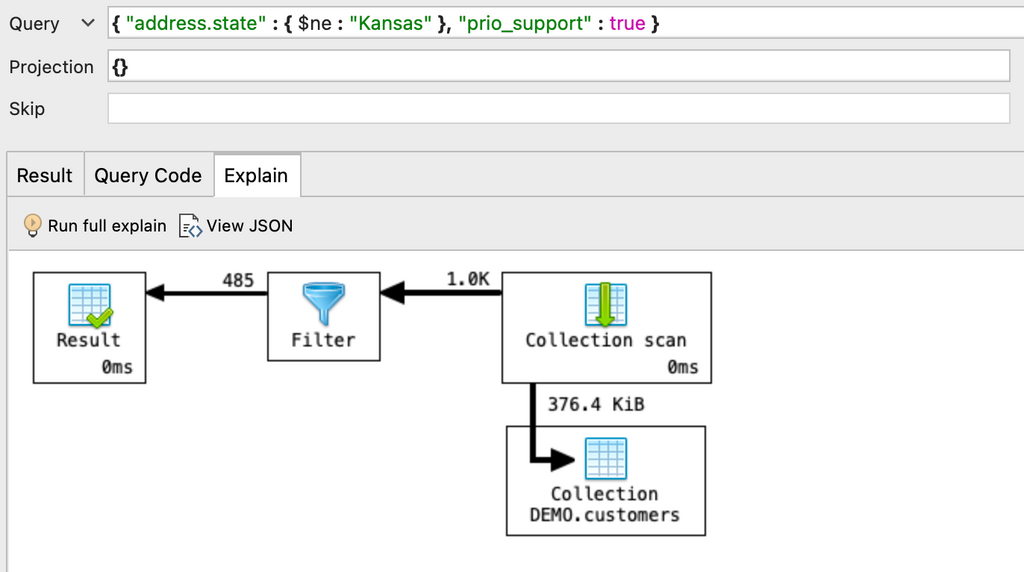
This is clearly way too large a number that could only point to an error in your query logic. (Do you spot the $ne
operator? 🙂 )
While this is a simple example, it still illustrates the usefulness of having a quick and visual way of double-checking the soundness of your query.
Brief vs. Full Mode
By default, Visual Explain shows the explain plan diagram in brief mode without runtime statistics, like this:
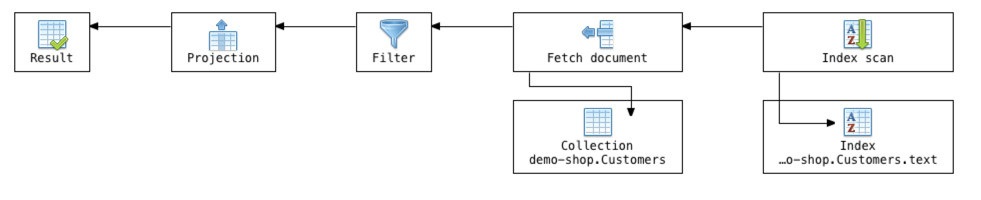
It’s fast and always available for all queries, without delay.
Full mode, on the other hand, shows both the stages and runtime statistics:
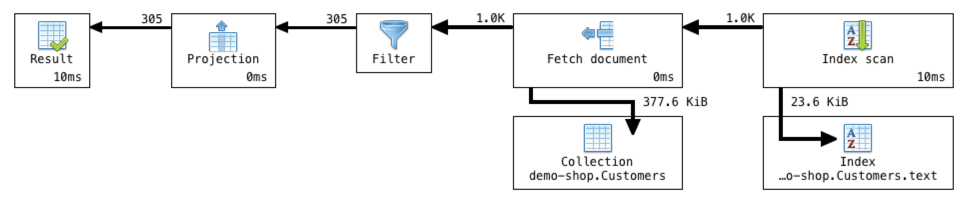
It requires running the query, which might cause a delay depending on its size.
To show full mode, click the Run full explain button in the Explain tab toolbar.
Hover over elements
You can hover over all elements in full mode to show additional help text, for example:
Arrows
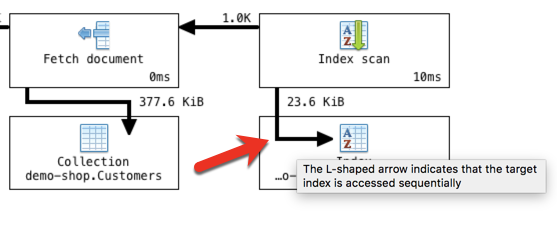
Runtime statistics
Hovering over runtime statistics displays information such as the estimated number of bytes processed by the stage:
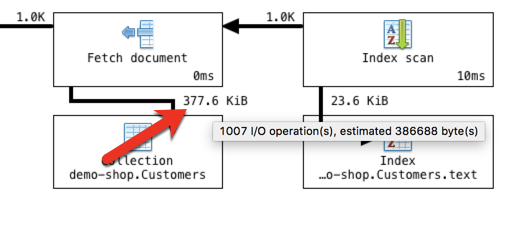
Or the full document count (for example, 1007 documents instead of 1.0K):
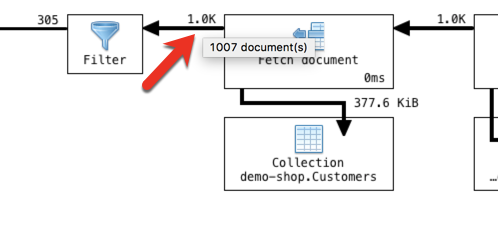
Or the time it took to execute an individual stage, or the whole query if you hover over the Result block:
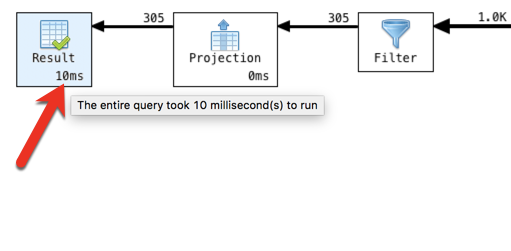
Query stages
Behind every MongoDB query is a query plan, and Visual Explain displays how this plan was executed, stage by stage.
View full description
To view a stage’s full description, right-click and select What’s this?.
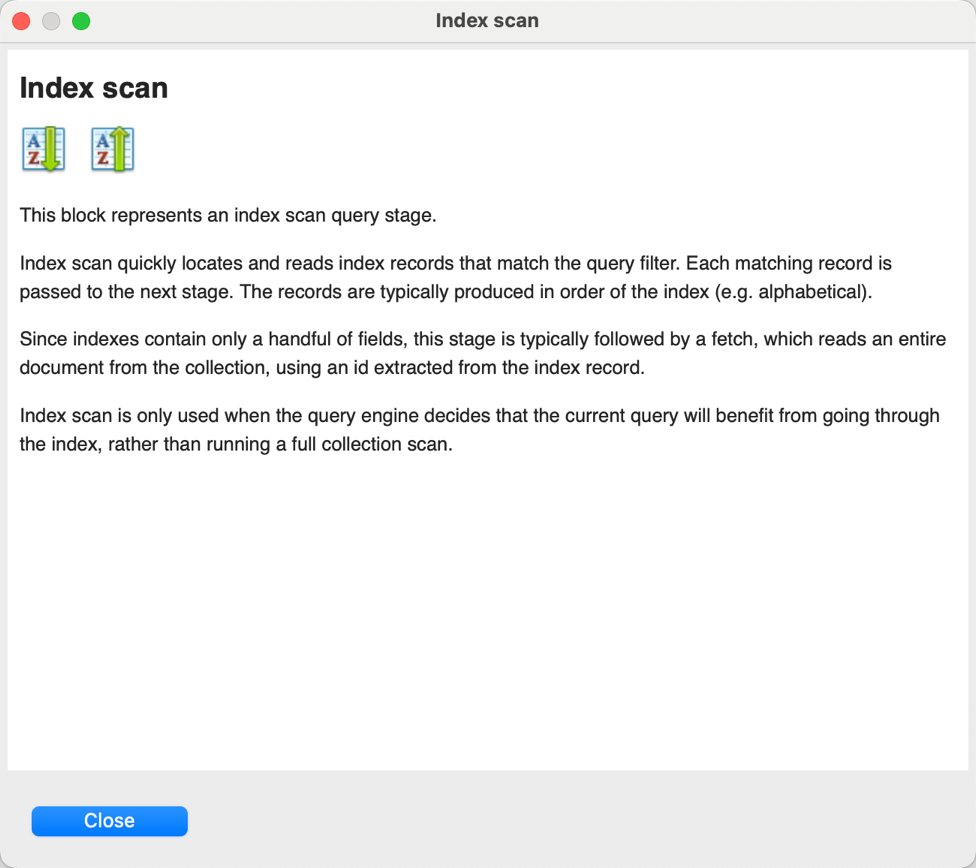
Runtime statistics
Visual Explain’s full mode displays runtime statistics, such as:
Stage output (number of documents produced)
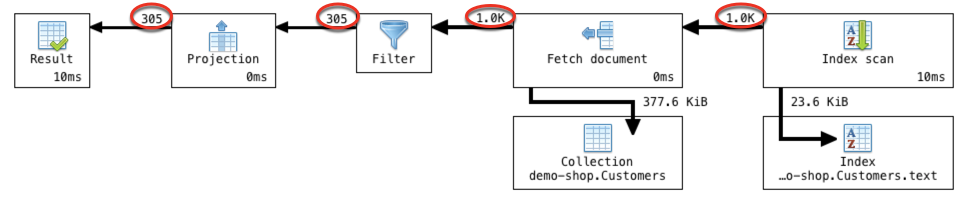
Shows the number of documents produced by a stage.
Execution time
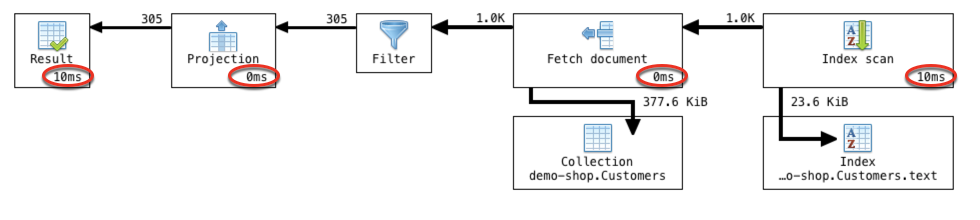
Tracks the number of milliseconds it took to execute a stage.
Amount of data processed
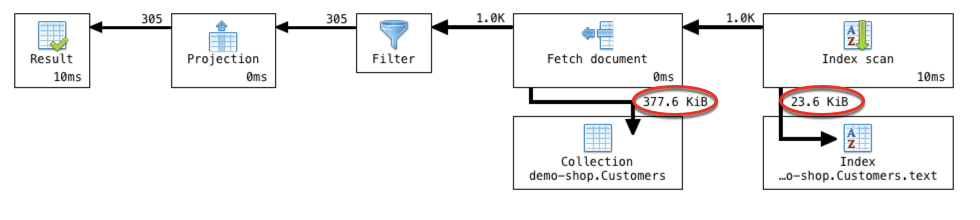
Displays the estimated amount of data processed to carry out the operation.
View the original query plan in JSON
You can view the query plan as a JSON document. Click on the View JSON button.
View a specific stage’s query plan in JSON
The original JSON document contains a lot of information not visible in the diagram, but it tends to be very nested and difficult to work with. To spare the back-and-forth scrolling for those who are interested in this extra information, Visual Explain makes it much easier to view a specific stage’s JSON fragment.
To show the extra information, right-click on a stage block and select View original JSON fragment.
Note that this displays only that stage’s JSON fragment and not the full query plan.
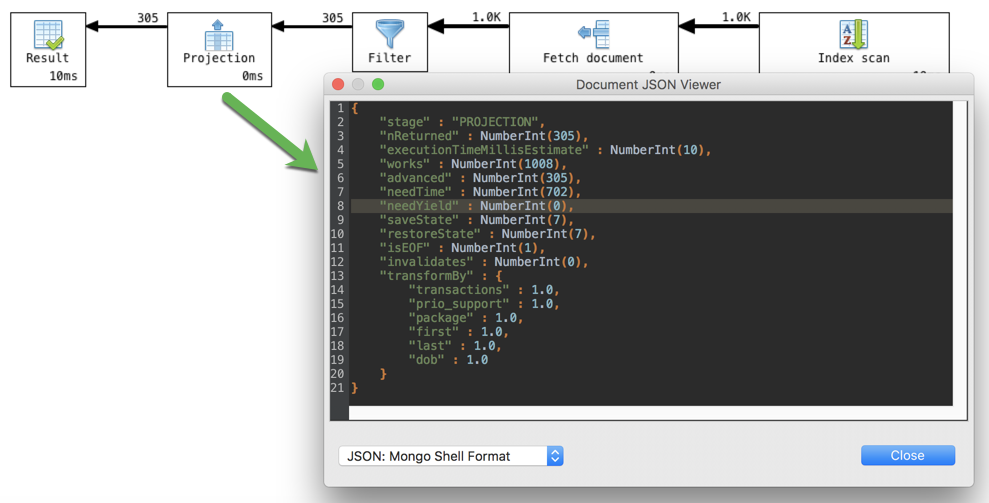
List of supported query stages
Represents a stage in an aggregation pipeline. All such stages read their input documents, apply operator-specific processing, and pass the result to the next stage.
Represents a collection scan query stage. Collection scan reads the documents from the collection one by one and passes them to the next stage. The documents are produced in no particular order. Collection scan is performed whenever the query engine decides that the easiest (or the only possible) way to get all the required documents is to read every document in the entire collection. Collection scan is a very I/O expensive operation, especially if the collection is large.
Represents a fetch document query stage. This stage reads a stream of ids produced by the previous stage. Each id then fetches a document with that id from the collection, then passes the document to the next stage. Although each individual document lookup is fast, this stage generates a lot of random I/O operations, which can become very I/O expensive, especially when multiplied by the number of documents.
Represents a document filter. Not a stage by itself, filtering is performed as a part of various stages. All the input documents are checked against some condition, and only those that match are passed to the next stage.
Represents an index scan query stage. Index scan quickly locates and reads index records that match the query filter. Each matching record is passed to the next stage. The records are typically produced in order of the index (e.g. alphabetical). Since indexes contain only a handful of fields, this stage is typically followed by a fetch, which reads an entire document from the collection, using ids extracted from the index record. Index scan is only used when the query engine decides that the current query will benefit from going through the index, rather than running a full collection scan.
Represents a union + deduplicate query stage. The purpose of this stage is to combine the results of its input stages, discarding identical documents that may be encountered more that once.
Represents a limit query stage. This stage reads the first N documents, passes them to the next stage, then terminates the process without examining the rest.
Represents a projection query stage. Projection transforms the input documents by removing some fields and possibly adding others. The transformed documents – which typically have just a small number of fields left – are passed to the next stage.
Represents a shard merge sort query stage. This stage combines the results obtained from several shards into one. All the shards’ results are assumed to be sorted, and the produced documents will also be sorted in the same order.
Represents a sharding filter query stage. This stage reads the input documents and passes through only those which satisfy the sharding condition for this shard. This way, a shard never produces a document that shouldn’t belong to it.
Represents a shard merge query stage. This stage combines the results obtained from several shards into one.
Represents a single shard query stage. This stage appears when all the documents to satisfy the query fall under the same shard, so it’s effectively a “merge” of one.
Represents a skip query stage. This stage reads the first N documents and discards them without producing anything, then passes the rest of the documents to the next stage.
Represents a sort buffer. Not a stage by itself, sort buffer limits the number of documents to be produced by the sort stage. This allows to reduce the amount of memory required for sorting.
Represents a sort key generator query stage. This is a preliminary stage to the sort stage that follows. From the input documents it extracts the fields required for sorting and passes the result to the sort stage.
Represents a sort query stage. Sorting rearranges the input documents and produces them in order. This stage can’t output anything before all the input documents have been processed, which potentially requires a lot of memory.
Represents a subplan stage. This stage plans each clause of the $or query individually, and then
creates an overall query plan based on the winning plan from each clause.
Represents a text match query stage. This stage returns only the documents that match the full-text search
expression.
Represents a text query stage. The purpose of this stage is to collect statistics on the full-text
search process itself.
This block indicates that a query stage of this type is not known
to the application, and is displayed as “just some stage”.
This block indicates that the format of the query plan is not supported and it is not parsed at all.
This article was originally published by Kathryn Vargas and has since been updated.