A collection in MongoDB is a group of documents, similar to a table in a relational database management system (RDBMS) or SQL database. MongoDB’s flexible schema allows documents within the same collection to have varying structures. This means that documents in a collection don’t need to contain the same fields and field data types can differ between documents.
In this guide, we’ll show you how to perform CRUD operations in the mongo shell compared to the Studio 3T GUI. We’ll also show you how to view MongoDB data in multiple collections side-by-side.
Create a MongoDB collection – db.createCollection()
In the mongo shell, you create a collection using the db.createCollection() method, with or without parameters.
Without parameters:
db.createCollection( "sea_mammals", )
With parameters, in this case “max” (maximum number of documents):
db.createCollection( "sea_mammals", { max: 300, } )
In Studio 3T, you can create a collection by right-clicking the target database or the target database’s Collections folder in the connection tree and selecting Add Collection.
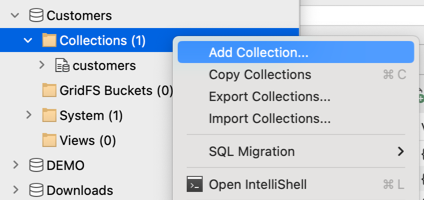
This opens the Add New Collection dialog where you can configure collation, validation, storage engine options, maximum number of documents, and more.
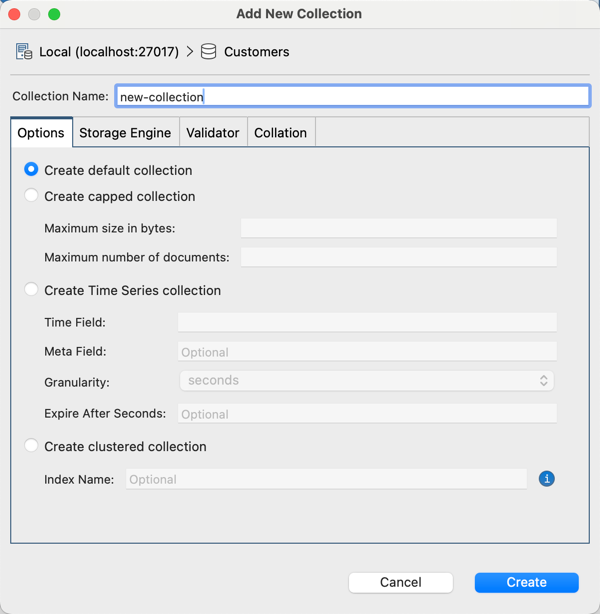
Drop a MongoDB collection – drop()
To delete an entire collection from the database, you use the drop() method:
db.manatees.drop( )
In Studio 3T, you can remove a collection by right-clicking the collection and selecting Drop Collection or pressing Del (Fn + Del).
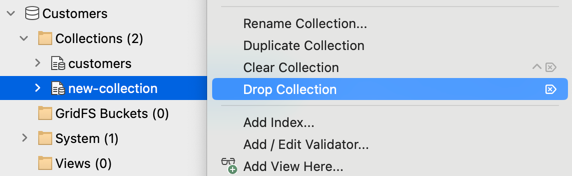
Clear a MongoDB collection
To delete all the documents from a collection, you can use the deleteMany() method without specifying any parameters:
db.manatees.deleteMany({})
In Studio 3T, you can delete the documents from a collection, while preserving index definitions and schema validation. Right-click the collection and select Clear Collection.
Rename a MongoDB collection – renameCollection()
To rename a collection, use the renameCollection() method in the mongo shell, specifying the target name in brackets:
db.sea_mammels.renameCollection( "sea_mammals", )
Or right-click the target collection and select Rename Collection in Studio 3T.
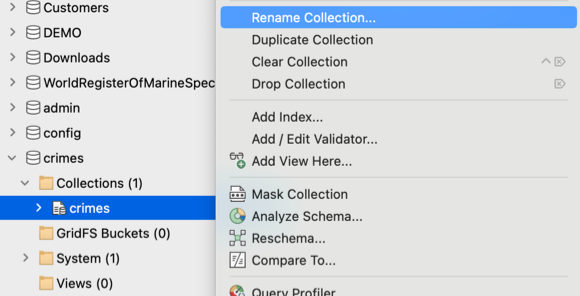
View a collection’s size – totalSize()
To determine a collection’s size, use the totalSize() method:
db.sea_mammals.totalSize( )
It returns the collection’s total storage size, plus the total size of each of the collection’s indexes in bytes.
In Studio 3T, you can see a collection’s size when you hover over the collection name in the connection tree.
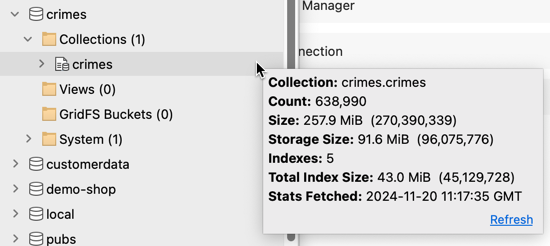
View a collection’s document count – count()
To count the number of documents in a collection, or the number of documents a find
query returns, use the count() method.
Total document count:
db.sea_mammals.count( )
Count for documents matching manatee
:
db.sea_mammals.count( { pet: "manatee", } )
In Studio 3T, you can see a collection’s document count when you hover over the collection name in the connection tree.
View a collection’s stats – stats()
To view a collection’s overall stats (including size and document count), use the stats() method. By default, size data is returned in bytes but can be modified with the scale
value, in this case kilobytes by specifying 1024
:
db.sea_mammals.stats( { scale: 1024, } )
In Studio 3T, you can view all these collection statistics at a glance by right-clicking the target collection and selecting Collection Statistics, which displays the information in tree view.
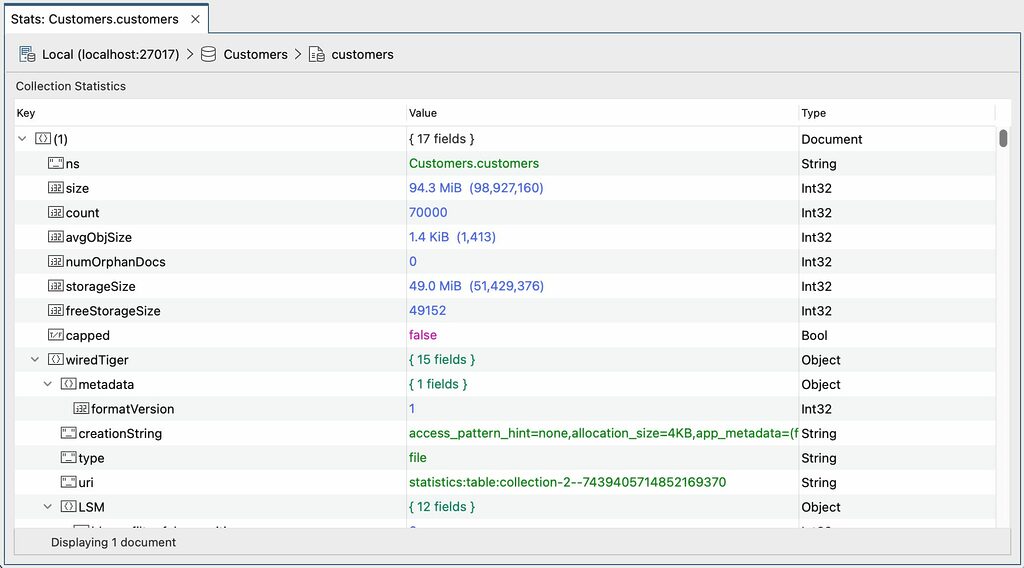
View multiple MongoDB collections side-by-side
Very frequently, you probably encounter one of these use cases:
- You want to easily compare test and production data sets.
- You simply want to look at and quickly compare result sets of different queries.
Let’s focus on the second use case and look at two separate views of the same collection.
This results in two different tabs displayed one after the other like this:
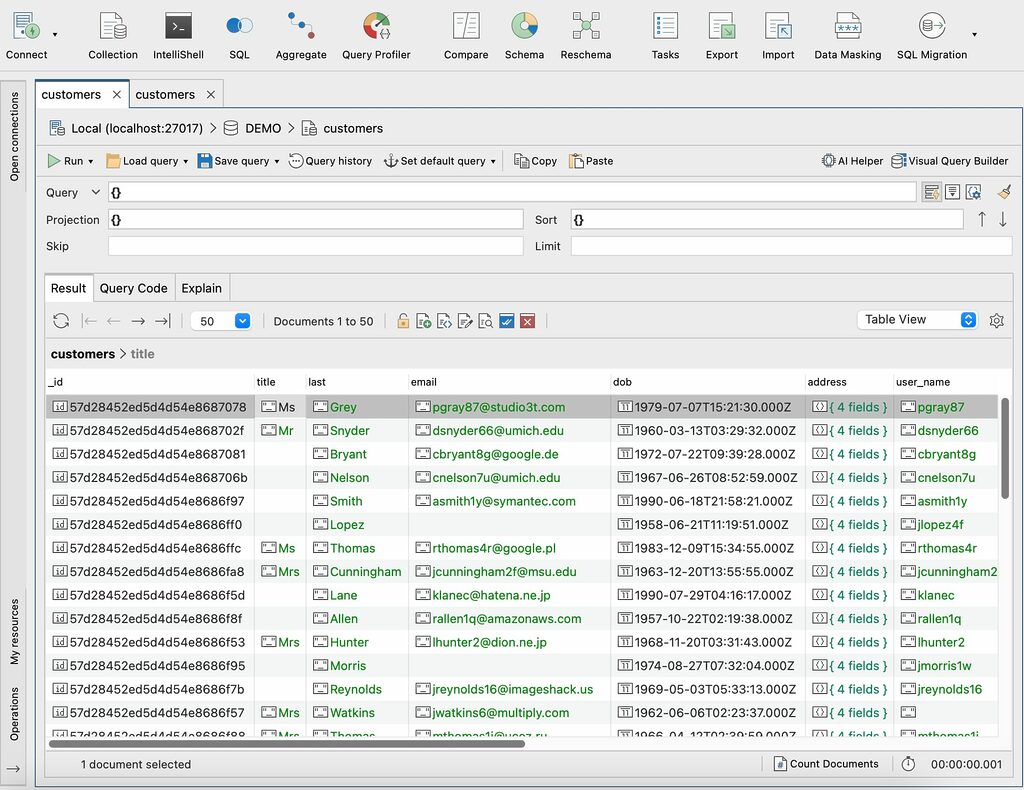
With the Split Vertically / Split Horizontally feature, Studio 3T allows you to quickly view collection datasets side-by-side (vertically) or horizontally.
Split vertically
Start by right-clicking the tab name at the top of any of the tabs and select Split Vertically.
Now your two tabs are shown side-by-side like this:
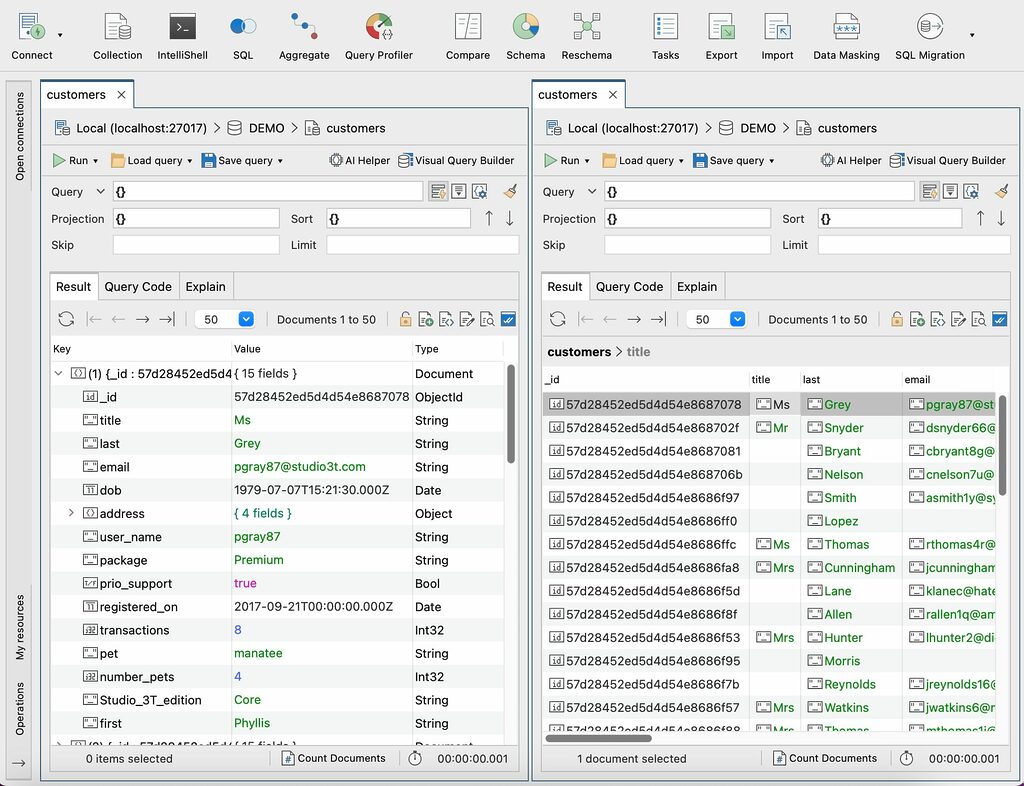
Split tabs horizontally
Or you could choose to split your view horizontally. Again, start by right-clicking one of your tabs and select Split Horizontally.
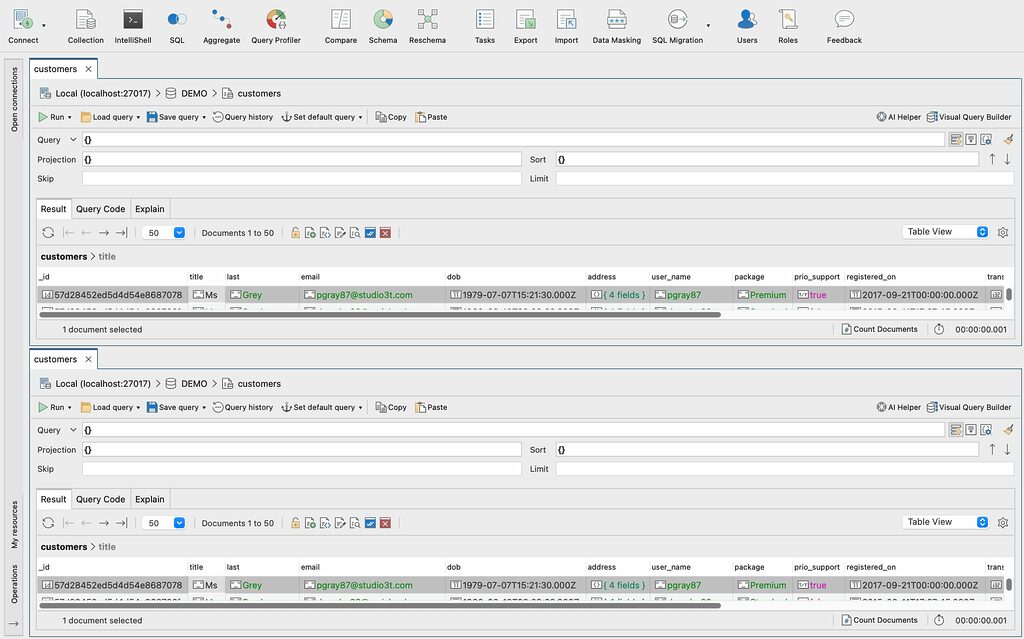
Drag and drop tabs
You can drag-and-drop tabs and reorder them if you have multiple tabs open and you want a quick way to compare them more easily.
Sort MongoDB collections
While in Studio 3T’s Table View, click a column header to sort your MongoDB data based on that column in ascending order.
If you click the column header again, the data is sorted in descending order.
Limit MongoDB collection size
By default, Studio 3T loads the first 50 documents of a MongoDB collection (equivalent to dbCursor.find({}).limit(50)) when first opening the Collection View.
As MongoDB documents can be up to 16MB in size, this can amount to serious traffic, so you can limit the initial number of documents for all collections or when you open the Collection View for a particular collection.
Limit number of documents loaded for all collections
- In Studio 3T > Preferences, go to Appearance and behaviour > Appearance.
- Locate the Initial page size when showing result documents setting.
- Change the number as needed and click OK.
Limit number of documents loaded for specific collections
You can also limit the initial number of documents loaded for a particular collection, which comes in handy when opening collections with exceptionally large documents.
- Right-click the collection you’d like to open in the connection tree.
- Select Open Collection with Custom Page Size.
- Enter the number of documents or initial page size you’d like to load initially and click OK.
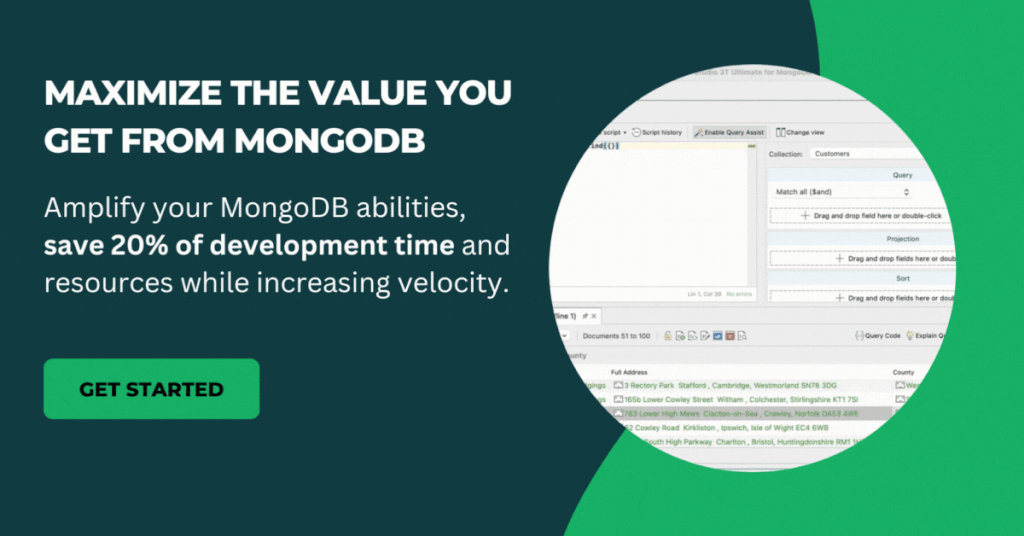
This article was originally published by Thomas Zahn and has since been updated.