A collection in MongoDB is a group of documents.
Collections in a NoSQL database like MongoDB correspond to tables in relational database management systems (RDBMS) or SQL databases. As such, collections don’t enforce a set schema, and documents within a single collection can have widely different fields.
In this guide, we’ll show you how to perform CRUD operations in the mongo shell and through the GUI, Studio 3T, as well as other time-saving shortcuts like copy-pasting collections across databases, viewing multiple collections at once, and more.
Create a MongoDB collection – db.createCollection()
In the mongo shell, creating a collection is done through the db.createCollection() method.
A collection can be created with or without set parameters, which include size (maximum size of a document in bytes).
Without parameters:
db.createCollection( "sea_mammals", )
With parameters, in this case “max” (maximum number of documents):
db.createCollection( "sea_mammals", { max: 300, } )
In Studio 3T, you can create a collection by right-clicking on the target database’s Collections folder from the Connection Tree and choosing Add Collection.
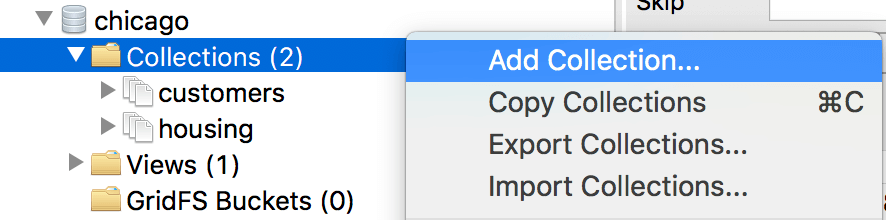
This will open a wizard where you can configure collation, validation, storage engine options, maximum number of documents, and more.
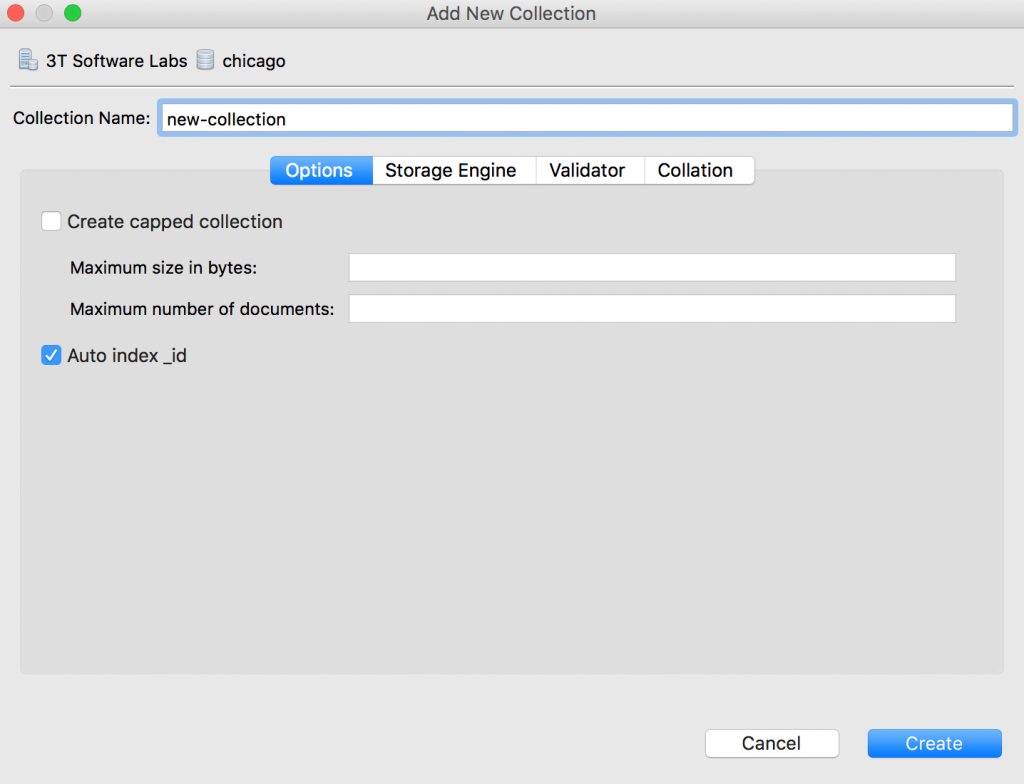
Drop a MongoDB collection – drop()
Removing a collection from the database is done through the drop() method. Leaving the field blank deletes the entire collection, like so:
db.manatees.drop( )
In Studio 3T, the same can be done by right-clicking on the collection and choosing Drop Collection or pressing Del (Fn + Del).
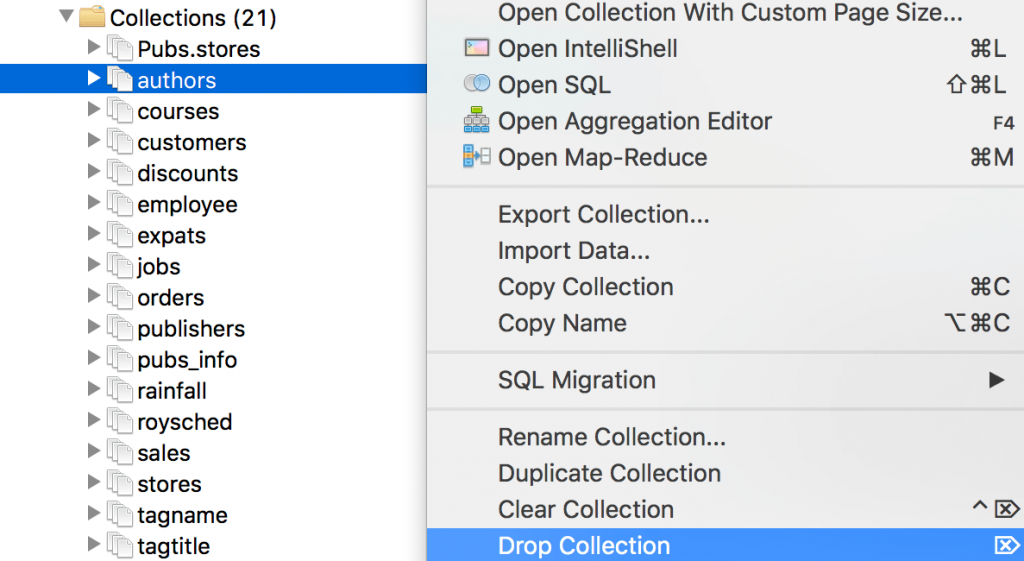
Rename a MongoDB collection – renameCollection()
Use the renameCollection() method in the mongo shell, specifying the target name in brackets:
db.sea_mammels.renameCollection( "sea_mammals", )
Or right-click on the target collection and choose Rename Collection in Studio 3T:
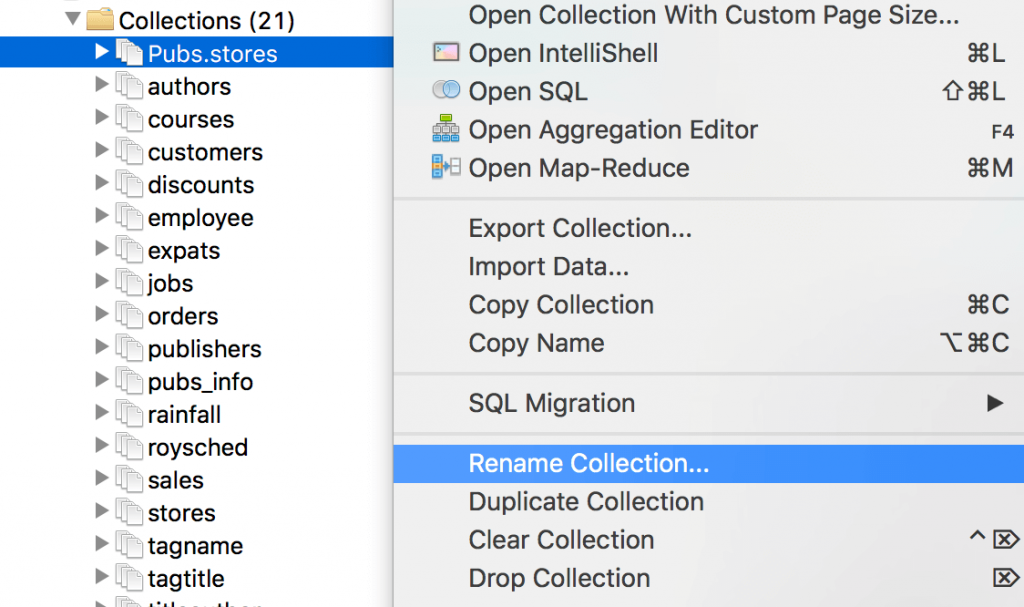
View a collection’s size – totalSize()
To determine a collection’s size, use the totalSize() method:
db.sea_mammals.totalSize( )
It returns the collection’s total storage size, plus the total size of each of the collection’s indexes in bytes.
View a collection’s document count – count()
To count the number of documents in a collection, or the number of documents a find
query would return, use the count() method.
Total document count:
db.sea_mammals.count( )
Count for documents matching manatee
:
db.sea_mammals.count( { pet: "manatee", } )
View a collection’s stats – stats()
To view a collection’s overall stats (including size and document count), use the stats() method. Size data by default is returned in bytes but can be modified with the scale
value, in this case kilobytes at by specifying 1024
:
db.sea_mammals.stats( { scale: 1024, } )
In Studio 3T, you can view all these collection statistics at a glance by right-clicking on the target collection and selecting Collection Statistics, which will display the information in tree view.
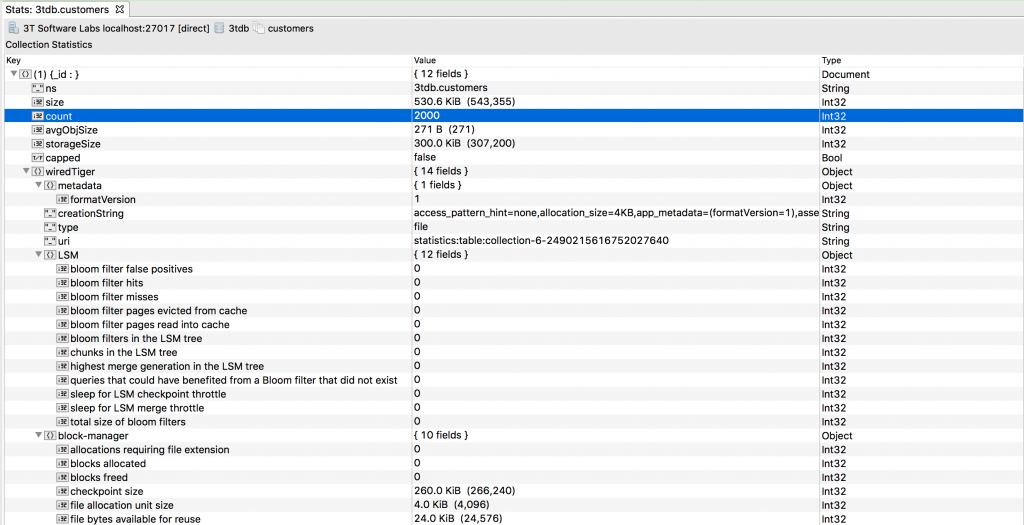
View multiple MongoDB collections side-by-side
Very frequently, you probably encounter one of these use cases:
- You want to easily compare test and production data sets.
- You simply want to look at and quickly compare result sets of different queries.
Let’s focus on the second use case and look at two separate views of the same collection.
This will result in two different tabs displayed one after the other like this:
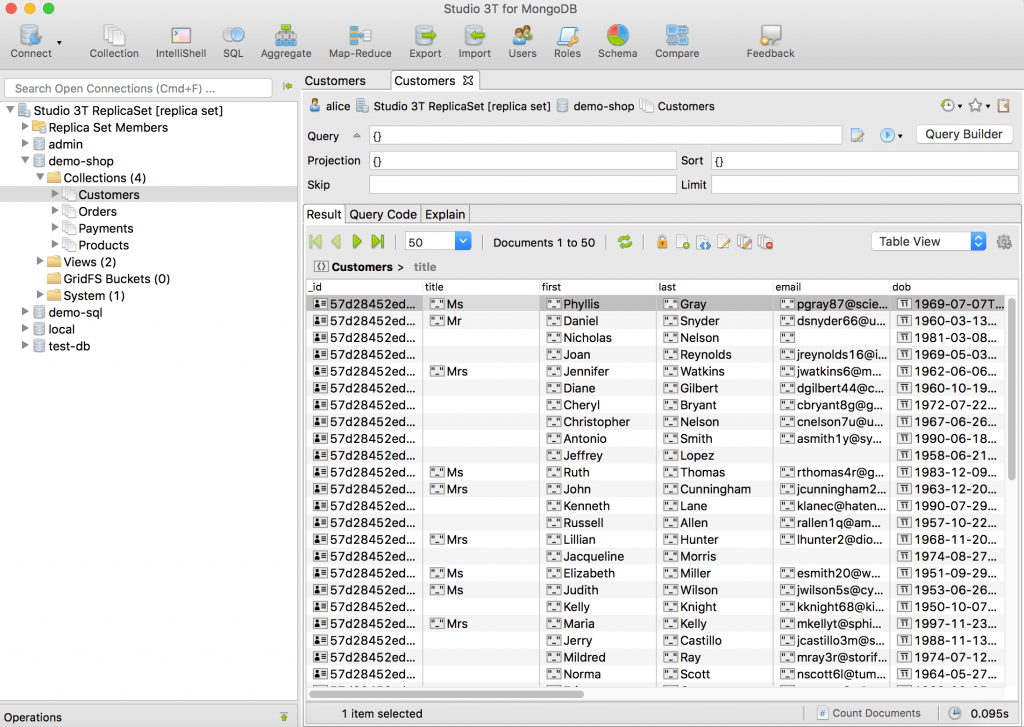
With the Split Vertically / Split Horizontally feature, Studio 3T allows you to quickly see, in a single glance, multiple tabs side by side. You can choose to display the two datasets side by side vertically or horizontally.
Split vertically
Start by right clicking on any of the tabs and choose Split Vertically.
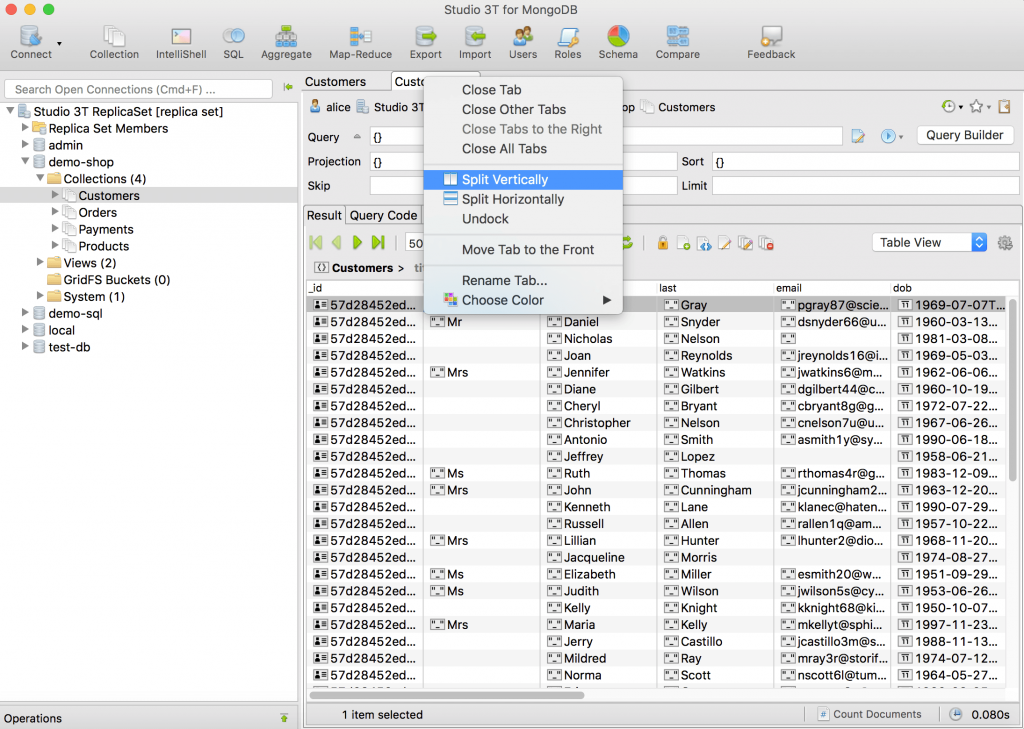
Now your two tabs will be shown side-by-side like this:
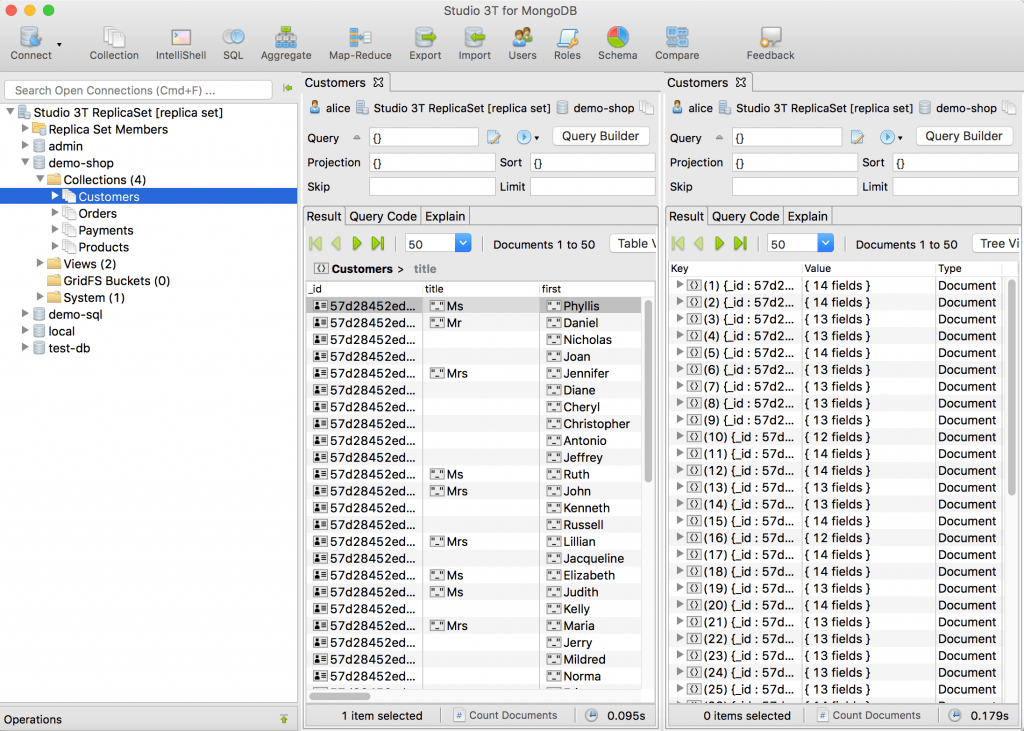
Split tabs horizontally
Or you could choose to split your view horizontally. Again, start by right clicking one of your tabs and now choose Split Horizontally.
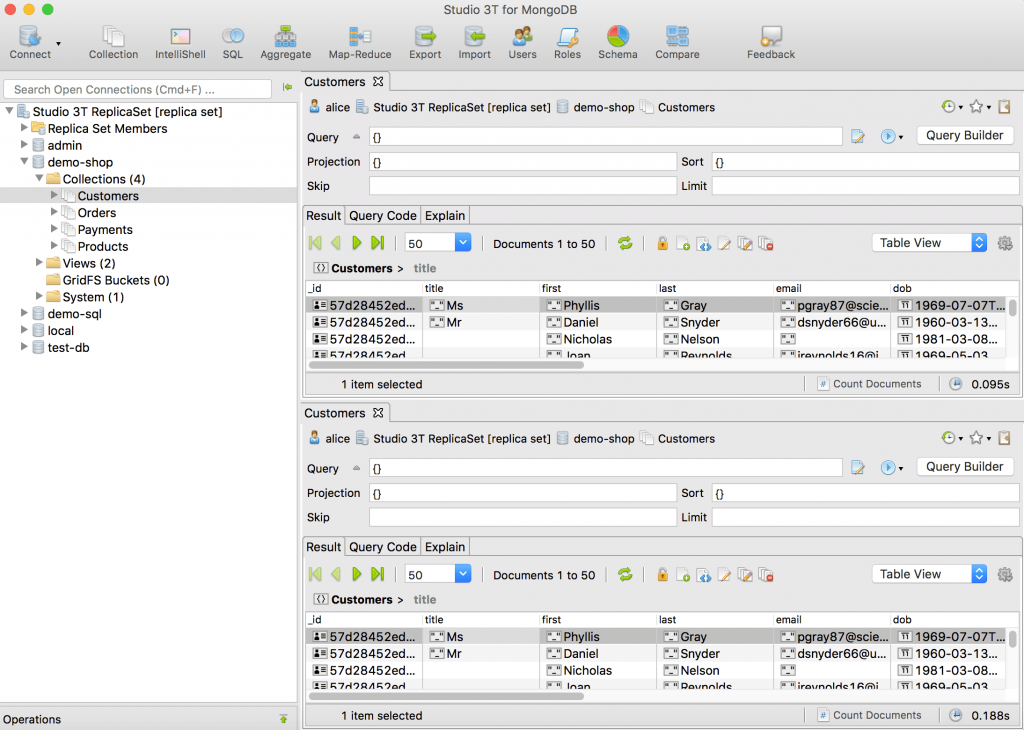
Drag and drop tabs
You can now even drag-and-drop tabs and reorder them in case you have multiple tabs open and you want a quick way to compare them more easily.
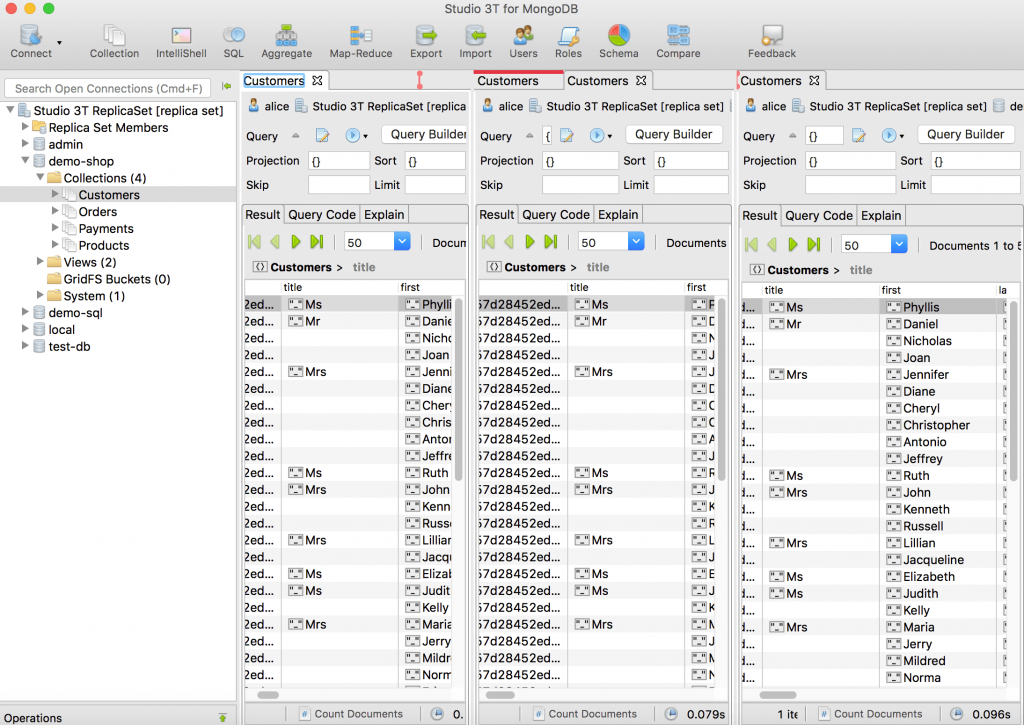
Sort MongoDB collections
While in Studio 3T’s Table View, tap on a column header once to sort your view based on the data in that column in ascending order.
If you click on the header again, the view will be sorted in descending order. Another tap will restore the original (unsorted) order.
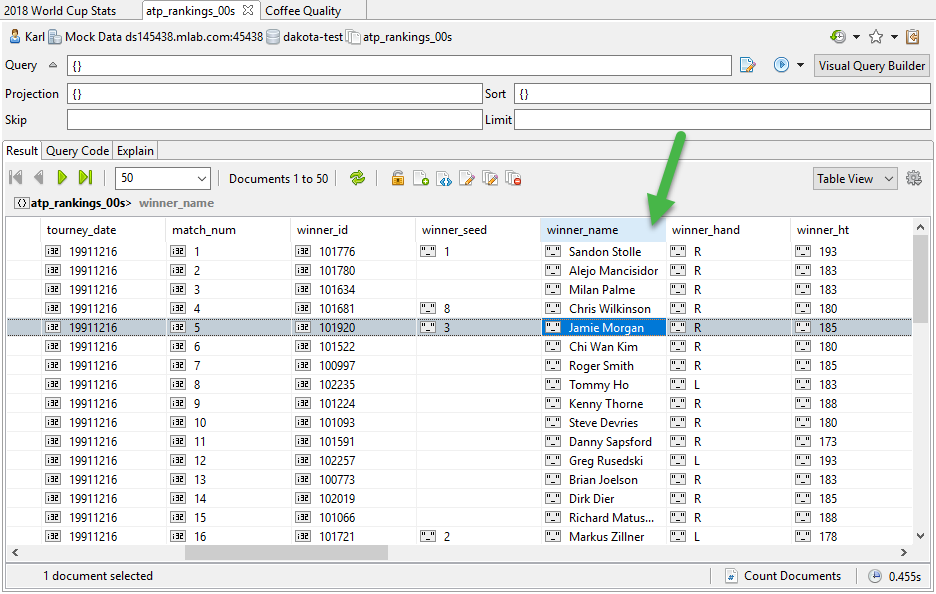
Limit MongoDB collection size
By default, Studio 3T loads the first 50 documents of a collection (i.e. dbCursor.find({}).limit(50)) when first opening a collection view.
As MongoDB documents can be up to 16MB in size, this can amount to serious traffic, so we’ve introduced a convenient way of limiting this initial number of documents either for all or specific collections.
Limit number of documents loaded for all collections
- Go to Studio 3T > Preferences.
- By default, you’ll land on the General tab.
- The first field you’ll see is Initial page size when showing result documents. Change the number as needed.
This will be the initial page size for all collections.
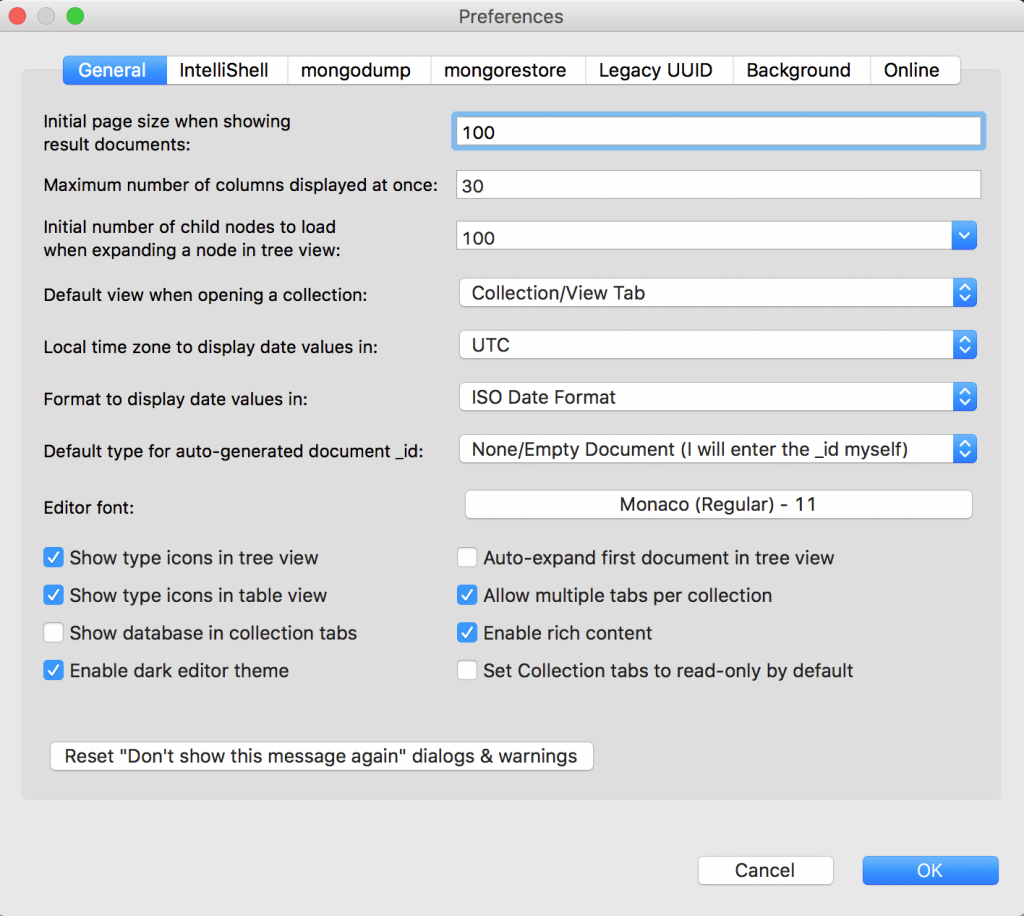
4. Click OK.
Limit number of documents loaded for specific collections
You can also limit the initial number of documents loaded for particular collections, which could come in handy when opening collections with exceptionally large documents, for example.
- Right-click on the collection you’d like to open in the Open Connections Tree.
- Choose the second option: Open Collection with Custom Page Size.
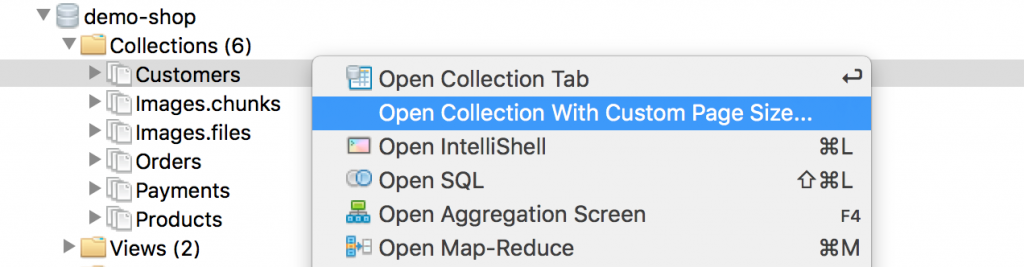
3. Enter the number of documents or initial page size you’d like to load initially.
4. Click OK.
Once you’ve defined your initial page size, explore your collections in Tree, JSON or Table View. Or for better data analysis, compare your MongoDB collections and spot differences in documents and fields.