Documents in a MongoDB database commonly include fields defined with the Array
data type.
A field configured with this type can contain zero or more elements that together form a list—or array—of values. The values might all be the same data type, or they might be different types.
For example, an Array
field might contain a mix of strings, integers, dates, embedded documents, or even other arrays.
When the documents in your collection contain arrays, you’ll likely want to build queries that can retrieve specific documents based on the values within those arrays.
To do so, you should understand the fundamentals of working with an array to ensure you can retrieve the documents you need when you need them.
In this section of the course, you’ll learn how to query a simple array in which all the values are configured with the String
data type.
To build and run these queries, you’ll use both IntelliShell and Visual Query Builder in Studio 3T. This will allow you to learn the basics of querying MongoDB arrays, while also seeing how easy it is to build these queries using Visual Query Builder.
Querying a MongoDB array
Throughout this course, you’ve been using the customers
collection to learn different concepts.
The documents in this collection contain the interests
field, which is an array that includes multiple String
values, usually between two and four.
Although the field can contain any number of values and those values can be different types, this particular collection is limited to only a few String
values.
The following figure shows a sample of the values in the interests
array, as they appear on the Result tab of the customers collection tab.
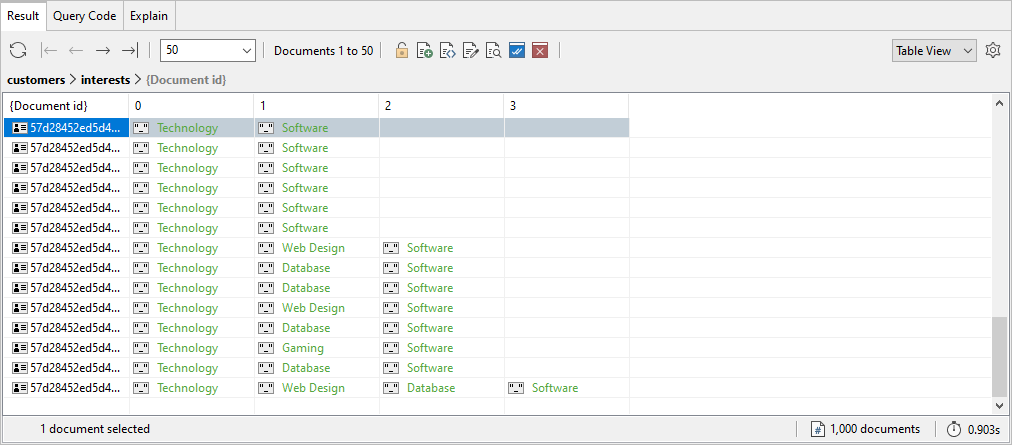
The figure provides a good cross section of the interests
array. Although most documents include the Technology
value, not all do, nor do the values appear in the same order.
If you want to retrieve documents based on a single value in the array, you can use the collection’s find
method, specifying the name of the array field and the target value, as shown in the following example:
use sales; db.customers.find( { "interests": "Gaming" } );
The statement calls the find
method on the customers
collection in the sales
database.
The method takes one argument, an embedded document that specifies the field name, followed by the value. In this case, the target field is interests
and the target value is Gaming
.
The query will return only documents that contain the Gaming
value in the interests
array. It does not matter where the Gaming
value falls within the array or how many values are included in the array. All that matters is that the array contains the specified element.
The MongoDB $elemMatch operator
When working with arrays, you might come across the $elemMatch
operator. You’ll certainly see it when using Visual Query Builder to create statements based on array values.
The operator can be used with the find
method or aggregate
method to return documents in which at least one element in the array matches the method’s specified search criteria.
For example, you can recast the previous statement to include the $elemMatch
operator, as shown in the following example:
use sales; db.customers.find( { "interests": { $elemMatch: {$eq: "Gaming"} } } );
The statement returns the same results as the preceding one, but with the added complexity that comes with the $elemMatch
operator.
The search condition in this case is the embedded document {$eq: "Gaming"
}, which means a document must contain the Gaming
value in the interests
array in order to be returned, just like the previous statement.
As the two examples demonstrate, you don’t need to use the $elemMatch
operator when specifying a single search condition.
The operator is mentioned here only because you’ll see it used in the code generated by Visual Query Builder, even when only one search condition is specified.
The next section of the course will cover the operator in more detail, where you’ll see examples that do benefit from the operator.
In the meantime, let’s consider how to build a query based on multiple array values, a process not quite as straightforward as a query based on a single value.
Suppose you want to return documents in which the interests
array contains both the Gaming
and Web Design
values. In an attempt to build such a query, you might try the following statement:
use sales; db.customers.find( { "interests": ["Gaming", "Web Design"] } );
Unfortunately, the query returns no documents from the customers
collection because it’s looking for those that are an exact match.
In other words, the interests
array must contain both the Gaming
and Web Design
values in the specified order and include no other array elements.
To return all documents that contain both values, you can instead use the $and
operator to define two search conditions, one for Gaming
and one for Web Design
, as shown in the following example:
use sales; db.customers.find( { "$and": [ { "interests": "Gaming" }, { "interests": "Web Design" } ] } );
The statement returns only those documents in which the interests
array contains both of the target elements. It does not matter what order they appear or whether the array contains other elements, as long the document includes both Gaming
and Web Design
.
If you want to return documents that include either value, you can instead use the $or
operator:
use sales; db.customers.find( { "$or": [ { "interests": "Gaming" }, { "interests": "Web Design" } ] } );
Now only one of the two conditions needs to be true for a document to be returned, although a document can include both values.
By the end of this section, you will learn how to:
- Use IntelliShell to query single and multiple values in an array
- Use Visual Query Builder to query a single array value
- Use Visual Query Builder to query multiple array values
What you will need:
- Access to a MongoDB Atlas cluster
- Access to the customers collection in the
sales
database