In this exercise, you’ll use IntelliShell to create and run several find statements that retrieve data from the customers collection.
To build and run the find statements
1. Launch Studio 3T and connect to MongoDB Atlas.
2. In the Connection Tree, right-click the sales database node and click Open IntelliShell. Studio 3T opens IntelliShell in its own tab in the main window.
3. On the IntelliShell toolbar, make sure Query Assist is enabled. This will make it easier to view the results returned by the mongo shell commands. Let’s also turn on auto-completion by clicking on the lightning bolt icon.
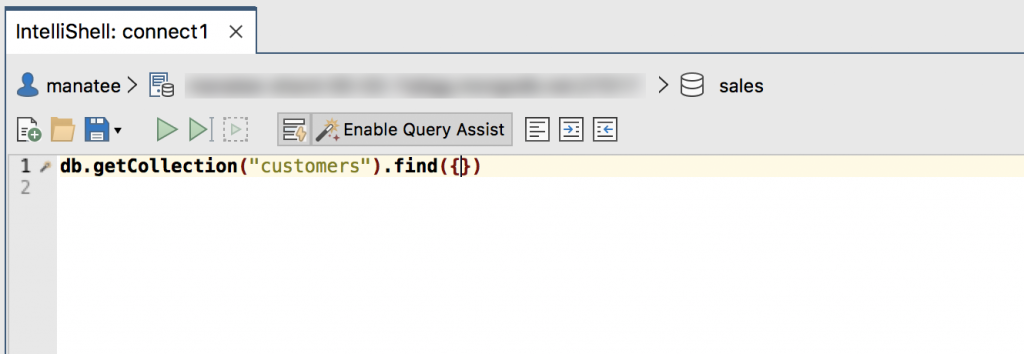
4. At the command prompt in the IntelliShell editor, type or paste the following command:
db.customers.find();
The command returns all data in the customers collection. The collection includes 1,000 documents, each one a customer record.
5. On the IntelliShell toolbar, click the Run entire script button ().
Studio 3T runs the find
statement and returns the results in the bottom window, displaying them in the Find tab. The tab is similar to the Result tab on a collection tab. For example, you can display the results in different views and work with the document data directly.
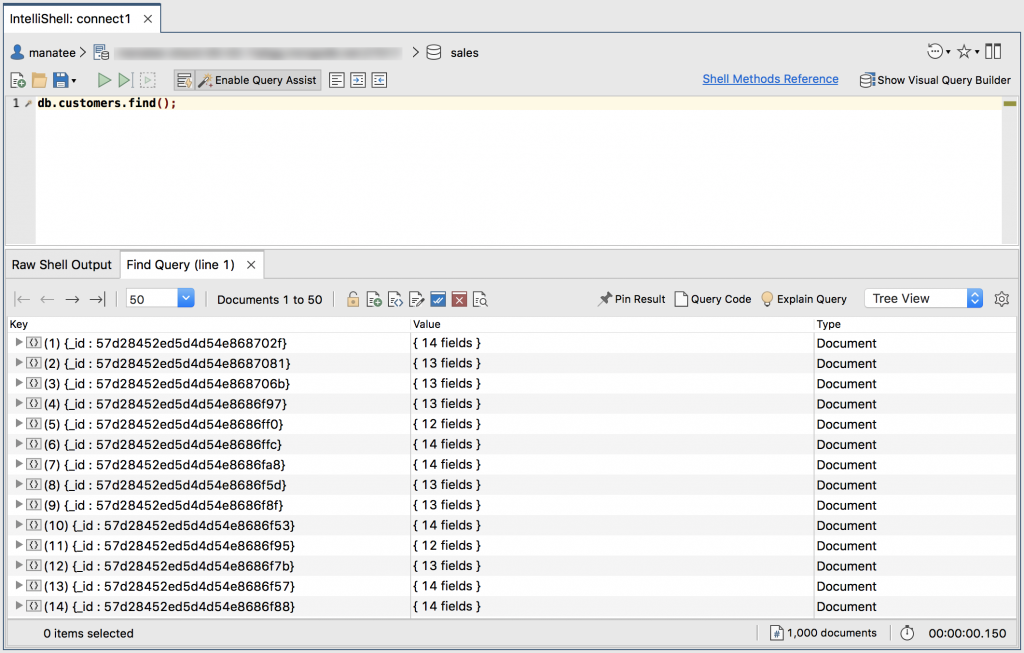
6. The next query you’ll create will limit the results to those documents whose device
field value is Apple iPhone
.
At the command prompt, delete the current statement and then type or paste the following command:
db.customers.find({"device":"Apple iPhone"});
The find
method includes a query argument with a single search condition. The search condition species that the device
field value must equal Apple iPhone
in order for a document to be returned. Notice that the search condition is enclosed in curly braces, with a colon separating the field name from its value.
7. On the IntelliShell toolbar, click the Run entire script button. The results should now include 537 documents.
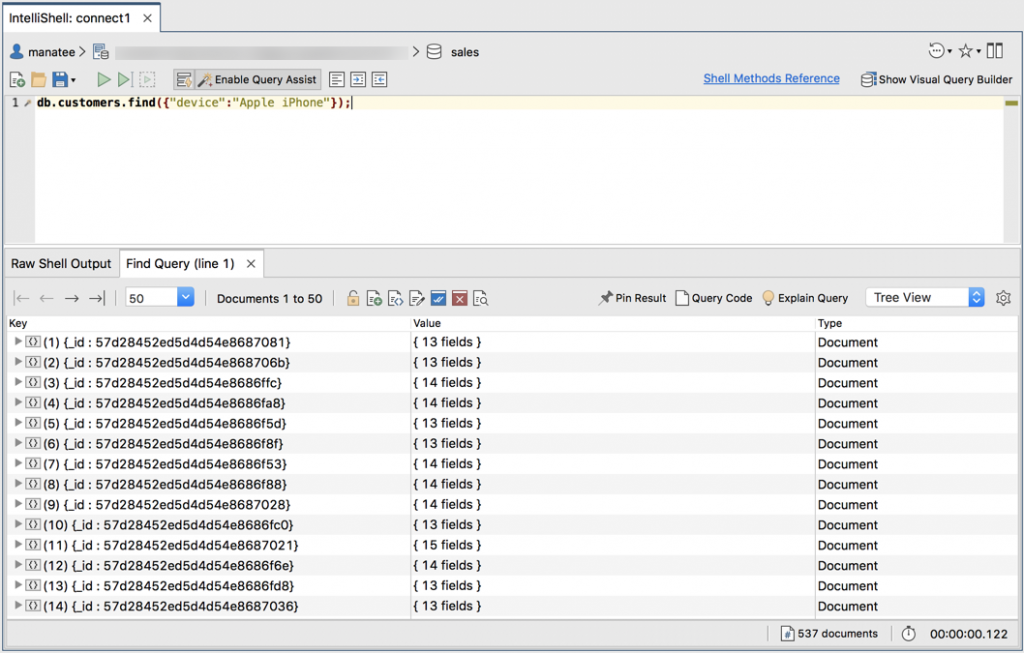
8. Next, you’ll create a query that limits the results to those documents whose address.state
field value is either Washington
or Oregon
. At the command prompt, delete the current statement and then type or paste the following command:
db.customers.find( {"address.state":{$in:["Washington", "Oregon"]}} );
As with the previous example, the query argument includes only one search condition.
In this case, however, the search condition uses the $in
operator to specify the field’s acceptable values (Washington
and Oregon
). Now only documents that include either of these values will be returned.
9. On the IntelliShell toolbar, click the Run entire script button. The results should now include only 29 documents—those whose address.state field value is either Washington
or Oregon
.
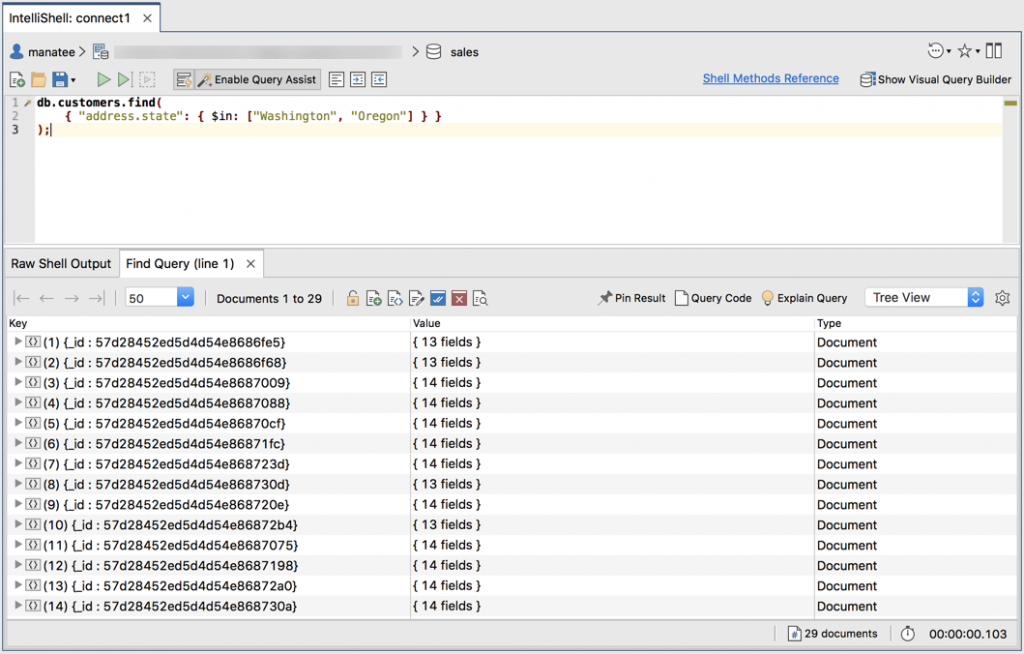
10. You’ll now combine the search conditions defined in the previous two examples, only this time you’ll specify that the device
field can include any value that contains the term iphone
.
At the command prompt, delete the current statement and then type or paste the following command:
db.customers.find( { "address.state":{$in:["Washington", "Oregon"]}, "device":/.*iphone.*/i } );
The first search condition specifies the permitted address.state
values, and the second search condition specifies the permitted device
values. You must separate the search conditions with a comma.
The value in the second search condition (/.*iphone.*/i
) is a regular expression that’s used for pattern matching.
- The forward slashes enclose the regular expression.
- The trailing i specifies that the search condition is case-insensitive.
- The two period/asterisk (.*) pairs indicate that zero or more characters can proceed or follow the term
iphone
.
As a result, the term can appear anywhere in the field’s value and still be included in the search results.
11. On the IntelliShell toolbar, click the Run entire script button. The results should now include only 14 documents.
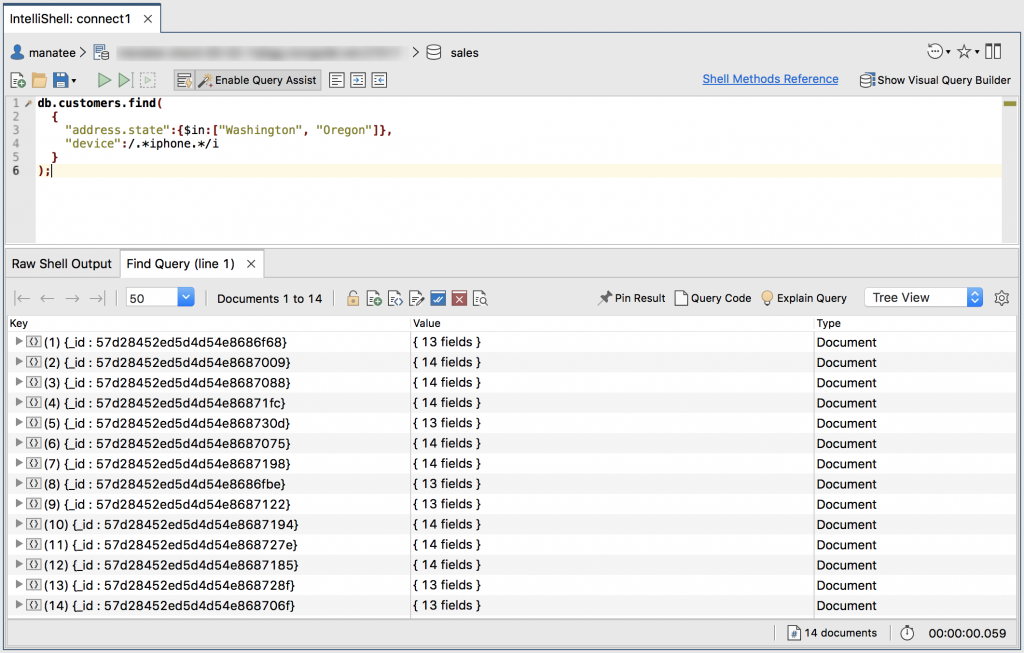
For each document, the address.state
field value is either Washington
or Oregon
, and the device field value contains the term iphone
.
12. The next step is to limit your search results to the first
, last
, and user_name
fields.
At the command prompt, delete the current statement and then type or paste the following command:
db.customers.find( { "address.state":{$in:["Washington", "Oregon"]}, "device":/.*iphone.*/i }, {first:1, last:1, user_name:1} );
The find
statement is the same as the preceding example except that it now includes a projection argument, which defines the three fields that should be returned in the results.
For each field, you must specify a value of 1
to indicate that the field should be included. When specifying multiple fields, you must separate them with a comma.
13. On the IntelliShell toolbar, click the Execute entire script button. The results should now be limited to the three specified fields (first
, last
, and user_name), as well as the _id
field, which is returned by default.
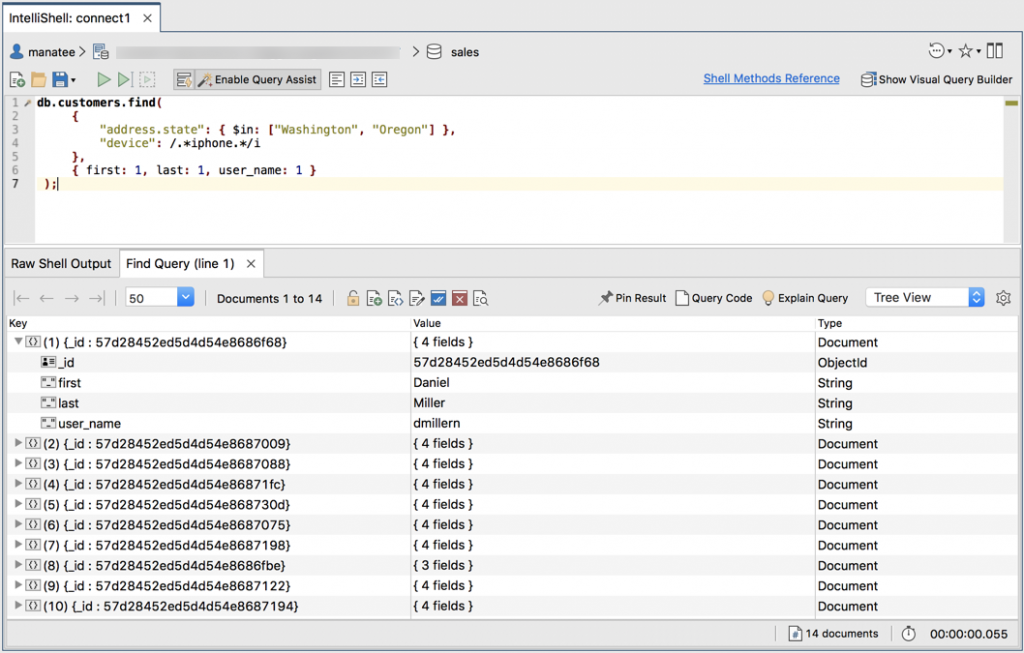
14. The final step is to update the query so the results are sorted by the last
values and then by the first
values. At the command prompt, delete the current statement and then type or paste the following command:
db.customers.find( { "address.state":{$in:["Washington", "Oregon"]}, "device":/.*iphone.*/i }, {first:1, last:1, user_name:1} ).sort({last:1, first:1});
To sort the results, you add a period after the find
method, followed by the sort
method.
The method’s arguments include the field names, specified in the order that the documents should be sorted. The value of 1
associated with the two fields indicates that the documents should be sorted in ascending order, rather than descending, which would take a value of -1
.
15. On the IntelliShell toolbar, click the Run entire script button. The results should look similar to the following figure.
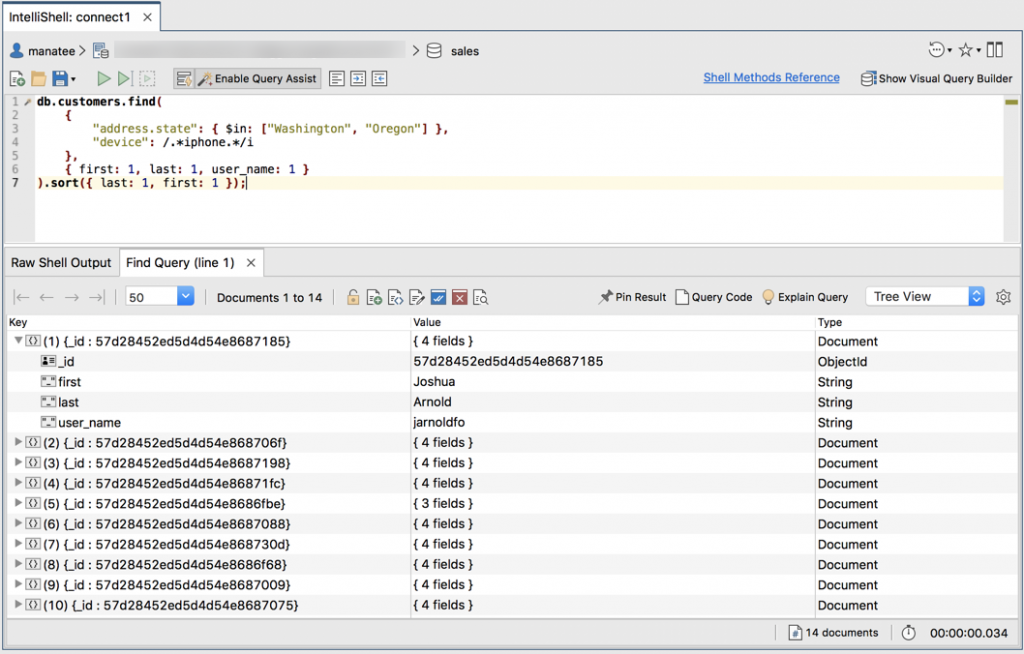
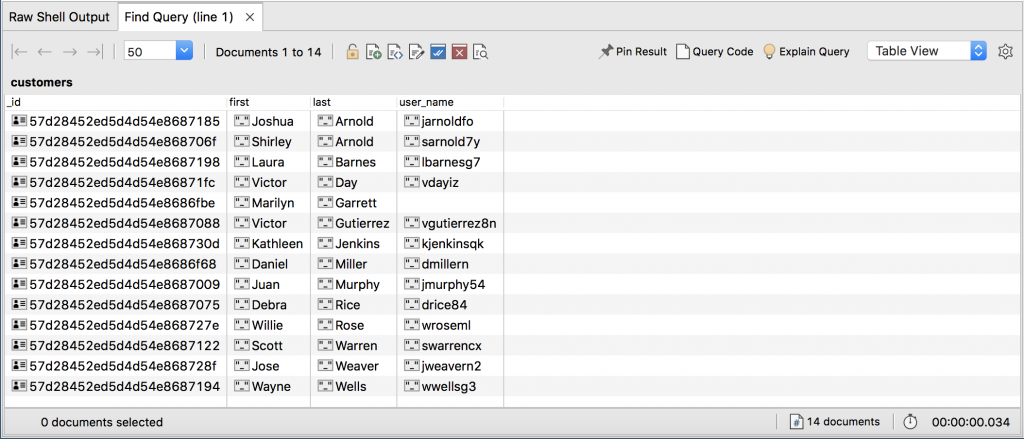
16. At the command prompt, delete the current statement but leave IntelliShell open for the third exercise.