In this exercise, you’ll use IntelliShell to add several documents to the customers
collection. Each document includes an Array
field that’s made up of several embedded documents. After you add the documents, you’ll use IntelliShell to run multiple queries based on values in the embedded documents.
To run the queries in IntelliShell
1. Launch Studio 3T and connect to MongoDB Atlas.
2. In the Connection Tree, right-click the sales database node and click Open IntelliShell. Studio 3T opens IntelliShell in its own tab in the main window.
3. On the IntelliShell toolbar, you’ll see that both the Auto-Completion button (the lightning bolt icon) and Query Assist button are already enabled by default. Make sure that both buttons are enabled. These will make it easier to view the results returned by the mongo shell commands.
4. At the command prompt in the IntelliShell editor, replace any existing code with the following insertMany
statement:
db.customers.insertMany( [ { _id: 1, first : "Maria", travel : [ { country: "Canada", visits: 3, rating: 7 }, { country: "Poland", visits: 1, rating: 8 }, { country: "Thailand", visits: 2, rating: 9 } ] }, { _id: 2, first : "Chen", travel : [ { country: "Thailand", visits: 3, rating: 7 }, { country: "Canada", visits: 2, rating: 9 }, { country: "Costa Rica", visits: 4, rating: 8 } ] }, { _id: 3, first : "Gladys", travel : [ { country: "Canada", visits: 1, rating: 8 }, { country: "Thailand", visits: 2, rating: 9 }, { country: "Australia", visits: 3, rating: 10 } ] } ] );
The statement adds three simple documents to the customers
collection. All three documents include the travel
field, an array made up of embedded documents.
Each embedded document lists a country that the customer has visited, along with the number of times the customer visited and how the customer rated the country, based on a scale of 1 through 10.
5. You now want to find those customers who have visited Australia three times. At the command prompt in the IntelliShell editor, replace the existing code with the following find
statement:
db.customers.find({ travel: { country: "Australia", visits: 3 } } );
The statement searches the travel
array to find documents with a country
value that equals Australia
and a visits
value that equals 3.
6. Execute this statement by clicking the Run Entire Script button. Studio 3T displays the results in the bottom window.
In this case, the query does not return any results even though the Gladys
document (the third document you added above) appears to match the search criteria.
The statement doesn’t return any documents because the search engine is looking for an exact match. The query specifies the country
and visits
elements, but not the rating
element, so an exact match is not possible.
7. To fix this, replace the existing code with the following find
statement:
db.customers.find({ travel: { country: "Australia", visits: 3, rating: 10 } } );
The new statement is just like the preceding one, except that it now includes the rating
element as a search condition.
8. On the IntelliShell toolbar, click the Run entire script button.
Studio 3T runs the find
statement and returns the results in the bottom window, displaying them in the Find Query tab. The query now returns the Gladys document.
Table View is selected by default. Switch to JSON View to view the document in full.
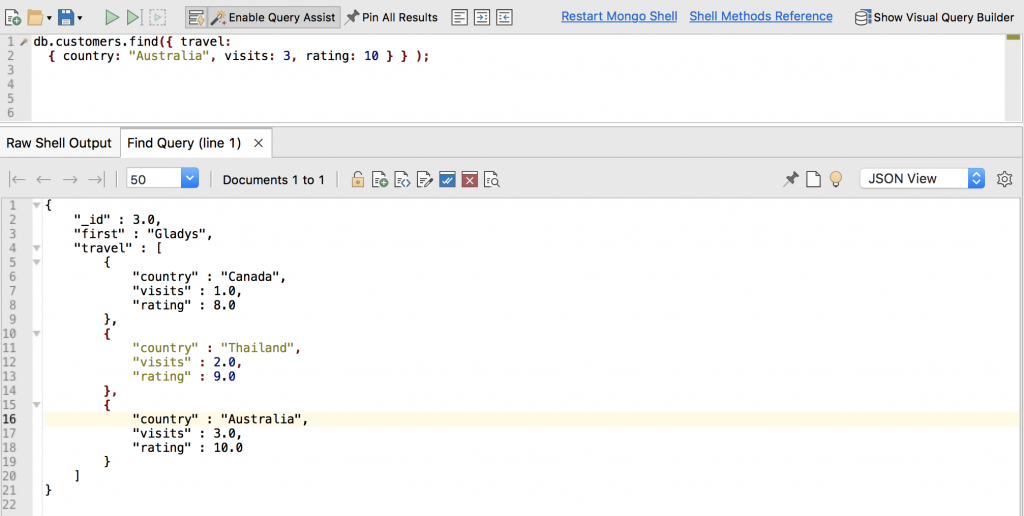
Although this approach works fine when you know the exact combination of fields and values, this is often not the case.
For example, the collection might include other customers who have visited Australia three times, but who have provided a different “rating”, in which case they would not be included in the results.
Another limitation with this approach is that you must specify the document elements in the exact order they’re saved to the database, or they will not be considered an exact match.
To test this out, return to the command prompt in the IntelliShell editor and replace the existing code with the following find
statement:
db.customers.find({ travel: { visits: 3, rating: 10, country: "Australia" } } );
The statement works just like the preceding one, except that the elements are in a different order.
9. Click the Run entire script button. As expected, the statement returns no documents because the element order is not an exact match.
10. Another approach you might take to find customers who have visited Australia is to recast the find
statement so that each search condition uses dot notation to specify the array and its elements.
At the command prompt in the IntelliShell editor, replace the existing code with the following find
statement:
db.customers.find({ "travel.country": "Australia", "travel.visits": 2 } );
Each search condition includes the array field name (travel
), followed by the specific element (country
and visits
, respectively). When you use the dot notation, you must enclose the fully qualified name in quotes.
For this statement, the visits
value is 2
, rather than 3
. This was done to help further explain issues that can arise when querying embedded documents.
11. Run the entire script. This time the statement returns the Gladys
document, even though she visited Australia three times, not two.
In this case, the query engine returns any documents that include a country
value of Australia
and a visits
value of 2, even if they’re in different embedded documents in the array.
To test this out further, you can change the field values used in the search conditions. At the command prompt in the IntelliShell editor, replace the existing code with the following find
statement:
db.customers.find({ "travel.country": "Canada", "travel.visits": 3 } );
The statement uses the same logic as the preceding one, only this time the country value is Canada
and the visits
value is 3
.
12. Click the Run entire script button. Now the statement returns all three documents, although Maria is the only customer to have visited Canada three times.
The problem this time is that all three documents include Canada as a country value, and all three include 3 as a visits value, even though they might not be in the same embedded document.
You have to be very careful constructing your queries when working with embedded documents. If this were an extremely large dataset, such a statement could return a massive number of documents, with few matching the intended criteria.
To get the results you want, you need to use the $elemMatch
operator.
13. At the command prompt in the IntelliShell editor, replace the existing code with the following find
statement:
db.customers.find( { travel: { $elemMatch: { country: "Canada", visits: 3 } } } );
Because the statement uses the $elemMatch
operator, the query engine will return only those documents in which at least one embedded document in the travel
array matches the specified search criteria.
14. Run the entire script. The statement returns only the Maria
document because it is the only one whose travel
array includes an embedded document with a country
value of Canada and a visits
value of 3
.
15. At the command prompt in the IntelliShell editor, replace the existing code with the following find
statement:
db.customers.find( { travel: { $elemMatch: { country: "Thailand", visits: 2, rating: 9 } } } );
Like the previous statement, this one also uses the $elemMatch
operator, only this time, it’s looking for customers who have visited Thailand twice and who have assigned a rating of 9 to the country.
16. On the IntelliShell toolbar, click the Run entire script button. The statement returns the Maria
and Gladys
documents because they were the only ones to meet the search criteria.
Although the Chen
document includes all these values, they are not within the same embedded document, so the document is not returned.
Note that you can achieve the same results without using the $elemMatch
operator:
db.customers.find({ travel: { country: "Thailand", visits: 2, rating: 9 } } );
This approach works only if you specify the elements in the exact order as they’re stored within the database and you match the specified elements exactly. Otherwise, the query engine will not return those documents.
The $elemMatch
operator ensures that you can retrieve the documents you want regardless of the number of fields or their order.
17. Leave IntelliShell open for the next exercise.