Let our Getting Started with MongoDB guide help you learn MongoDB, the most popular NoSQL database.
No introduction to MongoDB is complete without first looking at how it compares to SQL.
MongoDB vs SQL
Data organization
Instead of using tables, rows, and columns to establish relationships across data,
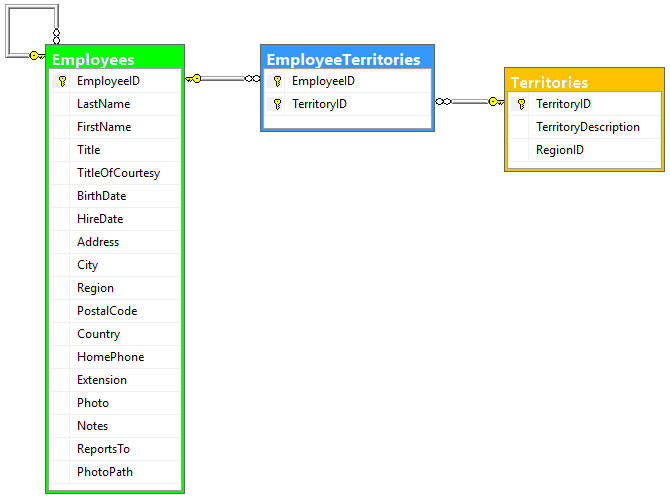
Source: SQL Blog
MongoDB uses name-value pairs or fields, which then make up documents, which make up collections.
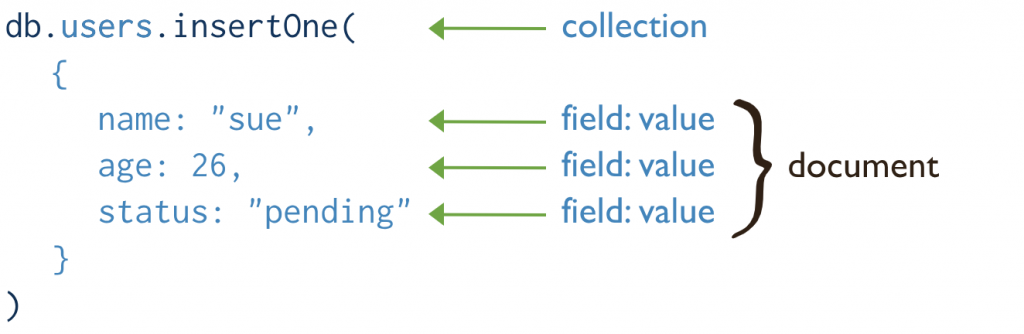
Source: MongoDB
Here’s a little cheat-sheet of how the database elements map to each other:
SQL | MongoDB |
---|---|
Database | Database |
Table | Collection |
Row | Document |
Column | Field |
MongoDB has two clear main benefits over SQL when it comes to data organization:
- Flexible and easy setup – Since a schema isn’t necessary upfront, getting started with MongoDB is quick and straightforward. You can dump unstructured data, query as you need, and worry about organizing your data later.
- Speed – Because data is stored in name-value pairs, querying large volumes of data can be quite fast in MongoDB compared to relational databases – with the right indexes.
Data scaling
You naturally collect more data as your project or business grows, and there are two ways of addressing this growth: vertical or horizontal scaling.
- Vertical scaling – Tethers you to a single server. As you deal with more data, you can add more storage space or upgrade to a more powerful CPU with higher RAM capacity to handle the workload, but you’re stuck with a server that might eventually prove inadequate for your current use case, or future use cases.
- Horizontal scaling – Spreads out the load over multiple servers instead of relying on a single high-speed, high capacity server.
MongoDB uses a form of horizontal scaling called sharding. It divides the data into different shards, so that each shard only contains a subset of the data. If you need more capacity, you can easily add more as you go.
When it comes to data scaling, MongoDB provides two obvious benefits:
- High scalability – You can distribute large volumes of data across multiple machines, and only add servers as needed.
- High availability – This is done in MongoDB through replica sets, or groups of mongod processes that maintain the same data sets. If a whole shard comes down, you are not going to be able to access the part of your data stored in it. However, if one or several nodes of the replica set of a sharded cluster comes down, you will still be able to access your data. Note that you can still set up a shard without implementing any replica set, with only one server.
Query language
SQL has Structured Query Language; MongoDB has the MongoDB Query Language. Sometimes referred to as MQL, it uses BSON (Binary JSON), which is based on JSON (JavaScript Object Notation).
If you’re proficient in JavaScript, you’ll crush it in the mongo shell, MongoDB’s interactive JavaScript interface. MongoDB commands can get clunky pretty quickly however, so features like query autocompletion can be a real time-saver.
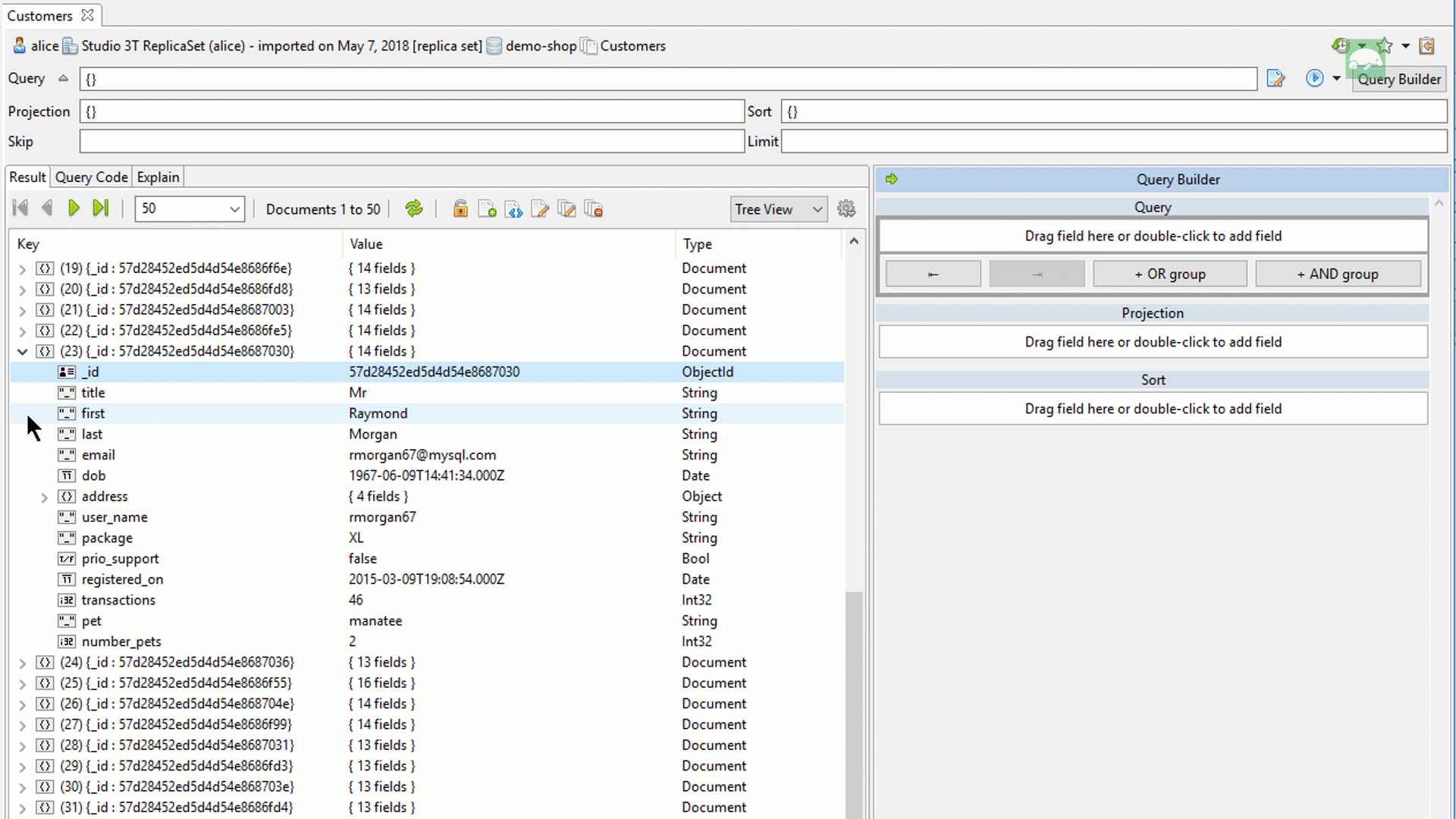
If you aren’t proficient in JavaScript but want to learn MongoDB, features like a drag-and-drop MongoDB query builder, SQL querying in MongoDB, or a MongoDB query generator from third-party tools like Studio 3T are an effective way of being productive from day one.
How MongoDB documents work
A document is the basic unit of data in MongoDB. It contains fields or name-value pairs.
A group of documents then makes up a collection.
Each MongoDB document requires a unique _id
field, which holds the ObjectId value. This serves as the primary key for each document. If this is missing, the MongoDB driver automatically creates it.
Fields
Within a MongoDB document, you can have fields of the following data types:
- Double
- String
- Object
- Array
- Binary data
- Undefined (deprecated)
- ObjectId
- Boolean
- Date
- Null
- Regular expression
- DBPointer (deprecated
- JavaScript
- Symbol (deprecated)
- JavaScript (with scope)
- 32-bit integer
- Timestamp
- 64-bit integer
- Decimal128
- Min key
- Max key
The maximum size of a document is 16 MB, but MongoDB uses GridFS to accommodate larger documents.
MongoDB documents also support arrays and embedded documents, and use the dot notation to access fields within them.
Arrays
Because data is stored flat in a MongoDB document, MongoDB can have fields with arrays – or a list of values – as values.
For example, the field marine-mammals
below has an array of three string values (manatee
, walrus
, seal
) as a value:
{ _id: “North America” marine-mammals: [ “manatee”, “walrus”, “seal”] }
Embedded documents
MongoDB allows you to embed documents within a document, to allow for the easier retrieval of information with just one query.
For example, take the two documents below. The first lists a specific marine mammal species and the continent it’s native to; the second lists the zoo(s) where one could visit this particular species.
{ _id: “Florida manatee” continent: “North America” }
{ species_id: “Florida manatee” zoo_address: { name: “ZooTampa at Lowry Park” street: "1101 W. Sligh Avenue", city: "Tampa", state: "FL", zip: "33604" } }
Instead of having to reference the second document every time you need the zoo location information, MongoDB allows you to embed the zoo_address
field from the second document as a subdocument of the first:
{ _id: “Florida manatee” continent: “North America” zoo_address: { name: “ZooTampa at Lowry Park” street: "1101 W. Sligh Avenue", city: "Tampa", state: "FL", zip: "33604" } }
Dot notation
It’s nice that MongoDB lets you use arrays and embedded documents, but how do you query them?
MongoDB uses dot notation to fetch values from elements of an array and fields of an embedded document.
Using the document above as an example, we can query the marine mammal database to find out exactly which species we can find in the zoo ZooTampa at Lowry Park
by writing the command:
db.marinemammals.find({"zoo_address.name" : "ZooTampa at Lowry Park"})
Most common MongoDB commands
Now that you’ve learned a bit about MongoDB, it’s time to hit the mongo shell and practice with the most common MongoDB commands for beginners.
Log into MongoDB
use dbName db.auth("userName", "password")
…making sure that the user exists in your current database.
Log out of MongoDB
db.logout()
List all databases
show dbs
Create a database (same as select a database)
use dbName
Show current database
db
Drop a database
After making sure it’s the right one:
db.dropDatabase()
Note that this command does not require the name of the database you are going to drop; it drops the current database in which you are in.
So, the first thing you must do is change to the database that you want to drop, this way:
use databaseName db.dropDatabase()
Create a user
The first step in adding a MongoDB user is to define the database where the user will be created (let’s take admin
):
use admin
Then, execute the following command:
db.createUser({ user : '<userName>', pwd : '<password>', roles : [ { role : '<roleName>', db : '<dbName>' } | '<roleName>', …] })
Drop a user
Again, choose the database from which you want to drop the user:
use dbName
And run the command:
db.dropUser('<userName>')
Assign a role to a user
As you might have noticed, it’s already possible to grant roles when adding a new user.
But in case you need to do it later, run the command:
use '<dbName>' > db.grantRolesToUser( '<userName>', [ { role : '<roleName>', db : '<dbName>' }, '<roleName>', … ] )
Revoke a role from a user
Run the following command:
use '<dbName>' db.revokeRolesFromUser( '<userName>', [ { role : '<roleName>', db : '<dbname>' } | '<roleName>' ] )
Create a collection
db.createCollection("collectionName");
Drop a collection
db.collection_name.drop()
Insert a single document in a collection
db.collectionName.insert({ field1: “value1”, field2: “value2” })
Insert multiple documents in a collection
db.collectionName.insertMany([{field1: "value1"}, {field1: "value2"}])
List documents in a collection
db.collectionName.find()
Update a single document in a collection
db.collection.updateOne(<filter>, <update>, <options>)
Update multiple documents in a collection
db.collection.updateMany(<filter>, <update>, <options>)
Delete all documents in a collection
db.collection.deleteMany()
if you want to delete all the documents in a collection using the ‘remove’ command, do not forget to use an empty document:
db.collection.remove({})
Delete all documents that match a condition
db.collection.deleteMany({ field1: "value1" })
or
db.collection.remove({ field1: "value1" })
Looking for another MongoDB tutorial?
Thanks for making it all the way to the end of our Getting Started with MongoDB tutorial. We hope it helped you get to know how the most popular NoSQL database works, and perhaps determine if it might be a suitable database for your next project.
The learning of course doesn’t end here. If you want to hone your MongoDB skills, we recommend these resources:
- Studio 3T – Not exactly a resource, but a MongoDB GUI and IDE with features that
enable anyone to use MongoDB, regardless of your knowledge level. - Academy 3T – Sign up for MongoDB 101, the free MongoDB course for beginners that covers the basics in just two hours
- MongoDB Tutorials – A roundup of the best MongoDB tutorials and courses, both free and paid
- Learn MongoDB – A list of MongoDB courses, books, YouTube videos, and more